Any programmer knows how vital variables are. Variables act as way-stations for coders/programmers where they pass information back and forth. Variables are generally crucial in computing since they enable code to be dynamic. In other terms, variables are referred to as information placeholders that change repeatedly depending on their usage. However, a significant problem that has affected most coders and programmers is keeping track of many variables in a project.
This tends to be a nuisance to most programmers in the field. There is one ultimate solution to this problem which is arrays. An array in programming is known as a data structure that stores elements with the same data type. Arrays are ideal in the storage of a collection of data. Arrays are of great importance to almost all coding languages, not forgetting scripting languages such as Bash. This article will concentrate more on associative array in Bash.
Shell scripting languages are known to offer users the ability to create, query, update, and manipulate indexed arrays. Don’t be confused by the term indexed arrays since it is a list of items that are prefixed with a number. The list plus the assigned number is wrapped in a variable, making it easy to present your code.
Associative array in Bash
The Bash scripting language has an added advantage since it can create associative arrays, and it treats the arrays as a normal array. An associative array’s primary function lets the user develop lists containing keys/indexes and values rather than just numbered values. The feature of associative arrays has been included in bash 4, meaning before we begin, we will look at the current version of Bash using the command line below:
bash --version
Output
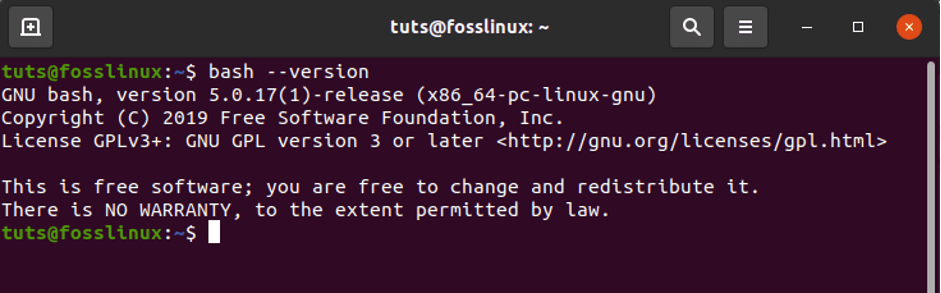
Bash Version
If your bash version is not version 4 and above, you will have to update it to work. Don’t be worried since the steps involved are straightforward. Bash can be updated on an Ubuntu server using the famous apt-get command. Some users might require to upgrade their Bash, and to do so, open your terminal and run the following commands:
sudo apt-get install --only-upgrade Bash
The command will update the bash shell to the newest version on your Ubuntu operating system. To ascertain that the update is successful, run the command above to check the installed/updated version of the bash shell (Bash –version). This command will print out a console with the current bash shell version on your Ubuntu server.
If, in some cases, you don’t have Bash installed in your Ubuntu server, don’t freak out. Run the commands below to install Bash:
sudo apt update sudo apt install bash
Declaration and Initialization of an Associative Array
In Bash, an associative array can be declared using the keyword ‘declare.’ Array elements, on the other hand, can be initialized during an array declaration or after variable declaration. The script below will create an array named assArray1, and the other four array values are individually initialized as indicated
declare -A assArray1 assArray1[fruit]=Mango assArray1[bird]=Cockatail assArray1[flower]=Rose assArray1[animal]=Tiger
Output
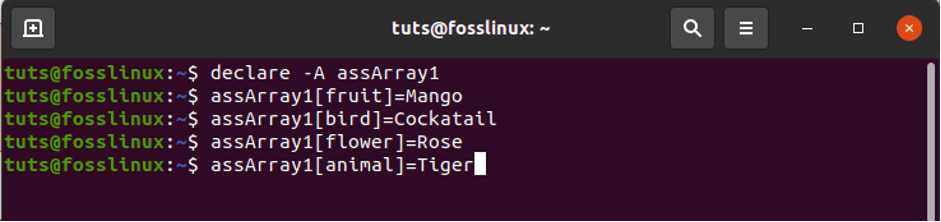
Example 1: Declaring and Initializing an Associative Array
During the array declaration, the following script named assArray2 will initialize an associative array. At the time of declaration, three array values with keys are defined.
declare -A assArray2=( [HDD]=Samsung [Monitor]=Dell [Keyboard]=A4Tech )
Output

Example 2: Declaring and Initializing an Associative Array
How to Access the Associative Array
There are two forms that a user can access an associative array. First, they can be accessed individually, and second, they can be accessed using a loop. Don’t get worried about the ways mentioned above since they will be covered in the tutorial. Please note that keys and values of an array can either be printed together or separately. By specifying the key value, the command line below will print two array values. We shall use the assArray1 to illustrate.
echo ${assArray1[bird]} echo ${assArray1[flower]}
Output
You will see a similar screen to the one below after running the command lines highlighted above.
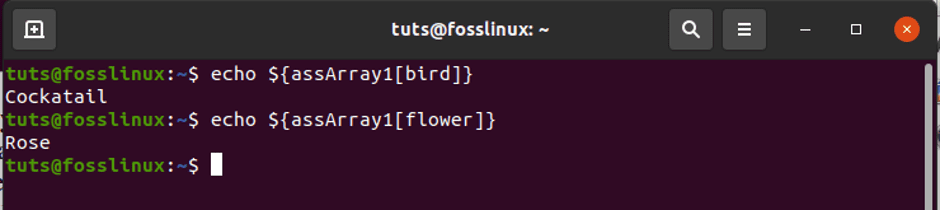
How to access the associative array
When printing, sometimes you will be required to print out all the keys and values of an array. This can be done using a bash perimeter expansion or a loop. First, let’s take a look at printing using a loop. If you have been to a programming class before, you must have come across this term severally. Execute the command below to print out all the keys and values of an array.
for key in "${!assArray1[@]}"; do echo $key; done
Output
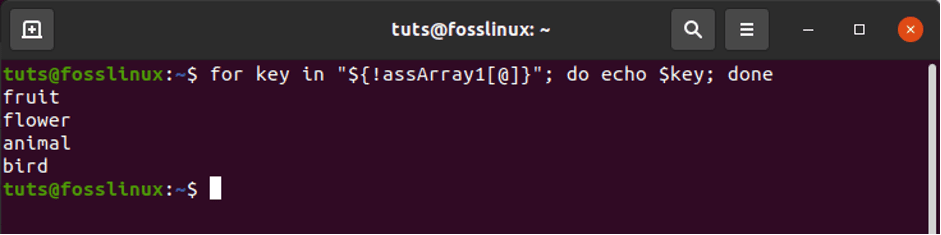
Example 1: Using loop to print out all the keys and values in an array
The following command will use the bash parameter expansion to print out all the keys and values of an array
echo "${!assArray1[@]}"
Output

Example 1: Using the bash parameter expansion to print out all the keys and values of an array
Note: the ‘!’ symbol in both of the commands is used to read the associative array’s keys.
Therefore, we have seen that all the array values can be printed using either a bash parameter expansion or a loop. Below is another example to elaborate on the use of the two methods. The first command will print the array values using a loop, whereas the second command will print out the array values using a bash parameter expansion.
for val in "${assArray1[@]}"; do echo $val; done
Output
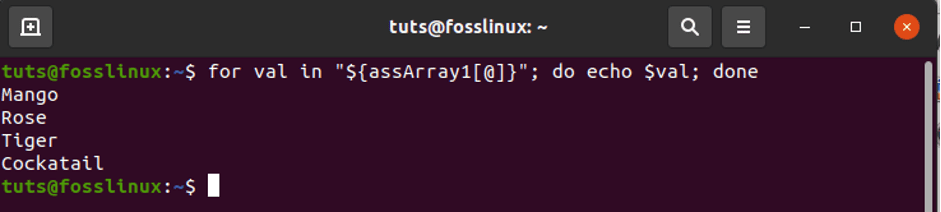
Example 2: Using loop to print out all the keys and values in an array
echo "${assArray1[@]}"
Output

Example 2: Using the bash parameter expansion to print out all the keys and values of an array
Note: the loop method can be used to print both keys and values of an associative array. For illustration, we will use our first array, assArray1. In this method, each array key has to be parsed in every step of the loop. The used key is then used as the array index that aids in reading the corresponding keys’ values.
Execute the command line below
for key in "${!assArray1[@]}"; do echo "$key => ${assArray1[$key]}"; done
Output
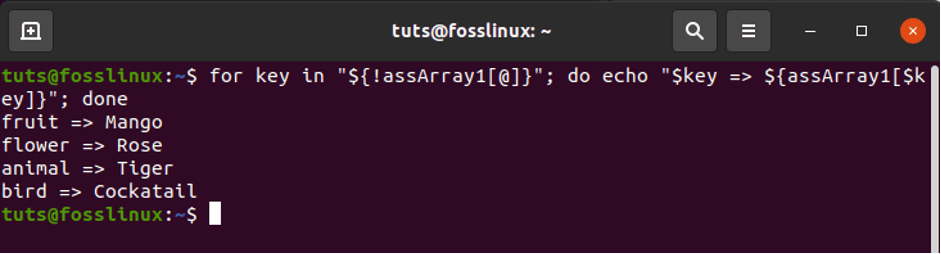
Using loop to print both keys and values of an associative array
How to Add Data to an Associative Array
After declaration and initialization of the array, adding new array elements to an associative array is possible. Using our second array, assArray2, a new value, “HP” and key “Mouse” will be added to the array, after which the current elements of the array will be rechecked. To complete the steps mentioned above, run the following commands
echo "${assArray2[@]}" assArray2+=([Mouse]=Logitech) echo "${assArray2[@]}"
Output
You will have a similar output to the one below after executing the commands above.
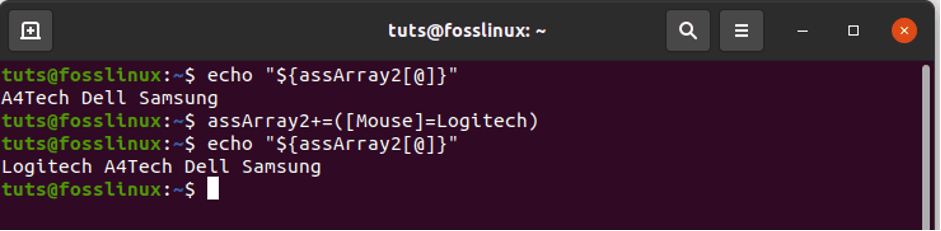
How to Add Data to an associative array
How to Delete Data From an Associative Array
Based on the key value, an element value in an associative array can be removed. When deleting data, the ‘unset’ command comes in handy. The command deletes particular data in an associative array. For instance, from our assArray2, let us delete the value that contains the ‘Monitor’ key. The command will check if the value has the key ‘Monitor.’ Then, with the unset command’s aid, the value will be deleted. The ‘echo’ command is used to check and ascertain whether the value has been deleted or not.
unset assArray2[Monitor] echo ${assArray2[Monitor]}
Output
If the command runs successfully, you will have a similar output to the highlighted below
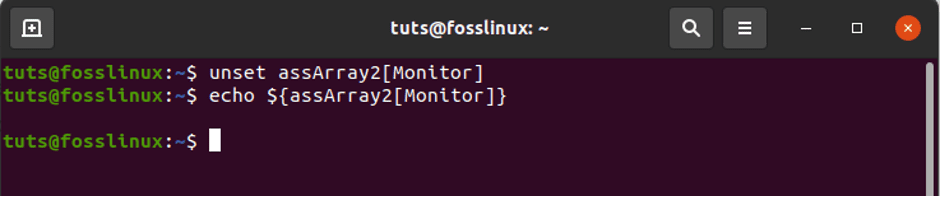
How to Delete Data From an Associative Array
How to Find a Missing Index in an Associative Array
A conditional statement, in this case, is vital since it helps in knowing if there is a missing index in an array. For instance, we can check for the array key ‘Monitor’ that we recently deleted. To do so, we shall run an if statement command to check if the key exists or not. Remember, we deleted the key from our previous example. Hence, the false value should be printed.
if [ ${assArray2[Monitor]+_} ]; then echo "Found"; else echo "Not found"; fi
Output
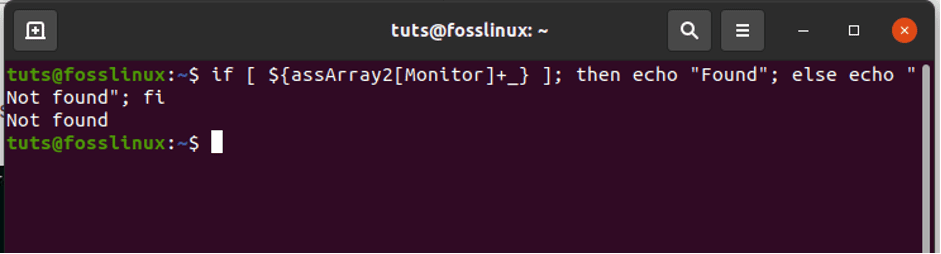
How to find a missing index in an associative array
How to Remove an Associative Array
The ‘unset’ command used to delete data from an associative array is also used to remove an associative array. In this example, we shall be using our first array, which is assArray1. The first command will print the values of the array. The second command containing the ‘unset’ command will remove the array. The final command will check if the array exists or not. If the array was successfully removed, then there will be nothing on the output console.
echo "${assArray1[@]}" unset assArray1 echo "${assArray1[@]}"
Output
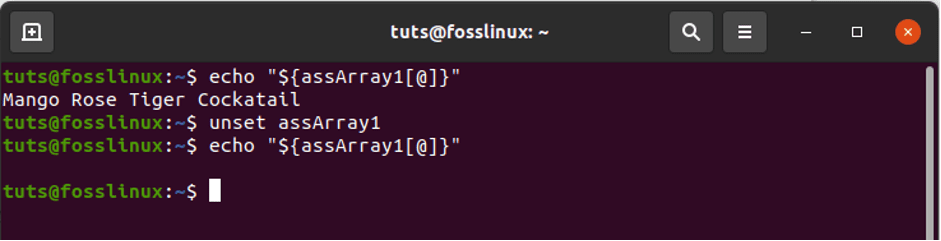
How to remove an associative array
Data Arrays
For the storage of related data, arrays are significant. You might be called upon to use variables to store data, but it is not the most appropriate way of storing and keeping track of large data tracks. Therefore, to keep your data more indexed and consolidated, you will then have to use arrays. Besides, the use of arrays to store and keep large track of data is more efficient than the use of variables. Arrays do not need an entry to exist like it is in variables. You should not be concerned about the exact amount of data to be stored before working on it. That makes arrays advantageous when dealing with extensive, unpredictable data. In short, arrays are much more potent than variables, and they are commonly used in most programming languages and scripting languages like Bash.
Conclusion
Using an associative array for data storage is vital when storing massive data containing key-value pairs in Bash. The article has covered how to declare and initialize associative arrays, parse array keys and values, add and delete array elements, remove an associative array and data arrays. Therefore, we hope all our readers will now be able to implement all the topics covered to improve their knowledge and understanding of associative arrays. Give it a try now.