Bash is a command-line shell and programming language widely used on Unix-based operating systems. It is an essential tool for system administrators and developers who need to automate tasks or perform complex operations. Bash provides various control structures such as if-then-else, for, and while loops that allow you to write scripts that perform different actions based on conditions, iterate through arrays or lists, and perform operations until a specific condition is met.
In this article, we will discuss the different Bash control structures and provide practical examples to illustrate their usage.
Writing efficient Bash scripts with control structures
If-then-else statements
If-then-else statements are one of the most basic Bash control structures. They allow you to perform different actions based on a condition or set of conditions. In Bash, if-then-else statements are written using the if, then, else, and fi keywords. The if keyword is used to specify the condition, and the then keyword is used to specify the action to be taken if the condition is true. The else keyword is used to specify the action to be taken if the condition is false. The fi keyword is used to mark the end of the if-then-else block. If-then-else statements are extremely useful in Bash scripting, as they allow you to automate tasks based on specific conditions or criteria.
The basic syntax of the if-then-else statement is as follows:
if condition then statement1 else statement2 fi
In this example, the script checks whether the condition is true or false. If it is true, statement1 is executed, and if it is false, statement2 is executed.
Here’s an example that checks if a file exists and prints a message accordingly:
#!/bin/bash if test -e "/home/fosslinux/Documents/my_backups/syslog_2.txt"; then echo "File exists" else echo "File does not exist" fi
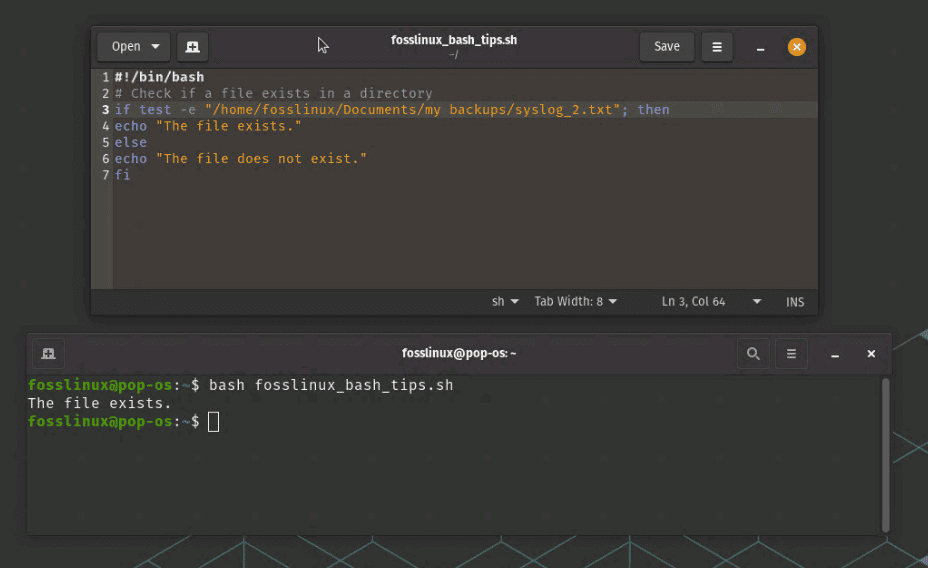
Using Conditional Statements
In this example, the script checks if the file exists using the -e option, and if it does, it prints “File exists”. Otherwise, it prints “File does not exist”.
For loops
For loops in Bash are used to iterate through a list of items, such as files in a directory, and perform a set of actions for each item in the list. The basic syntax for a Bash for loop is for var in list; do commands; done. The var variable is set to each item in the list and the commands are executed for each item. In Bash, list can be a range of numbers, a list of filenames or directory names, or a list of strings separated by spaces. For loops can be extremely useful in automating tasks that involve iterating through a large number of items, such as renaming files or processing data files. By using For loops, you can write more efficient and effective scripts that can save you time and effort in your daily tasks.
The basic syntax of the for loop is as follows:
for variable in list do statement done
In this example, the script iterates through the items in the list, assigns each item to the variable, and executes statement for each item.
Here’s an example that prints the numbers from 1 to 5:
#!/bin/bash for i in 1 2 3 4 5 do echo $i done
In this example, the script iterates through the numbers from 1 to 5 and prints each number using the echo command.
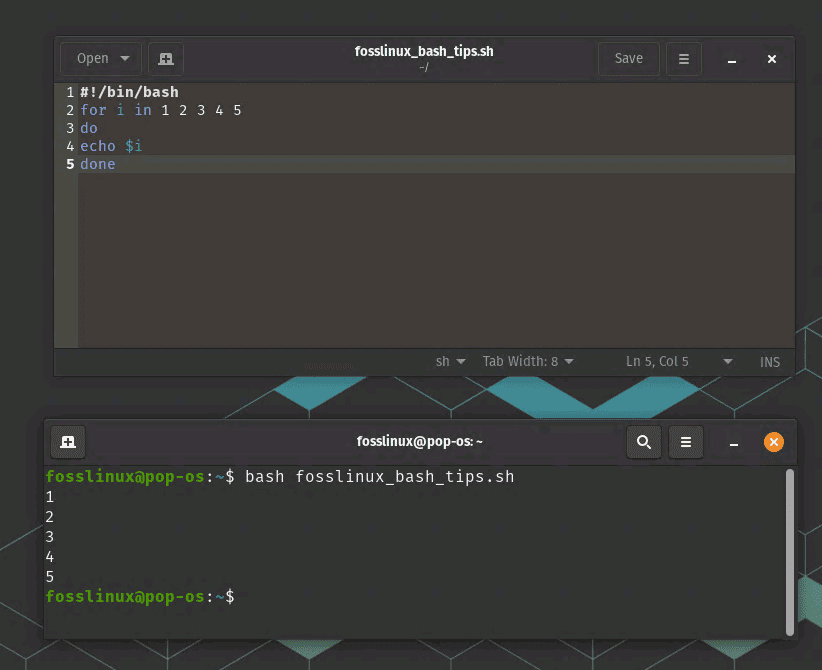
For Loops example
You can also use the for loop to iterate through the items in an array:
#!/bin/bash array=("item1" "item2" "item3") for i in "${array[@]}" do echo $i done
In this example, the script iterates through the items in the array and prints each item using the echo command.
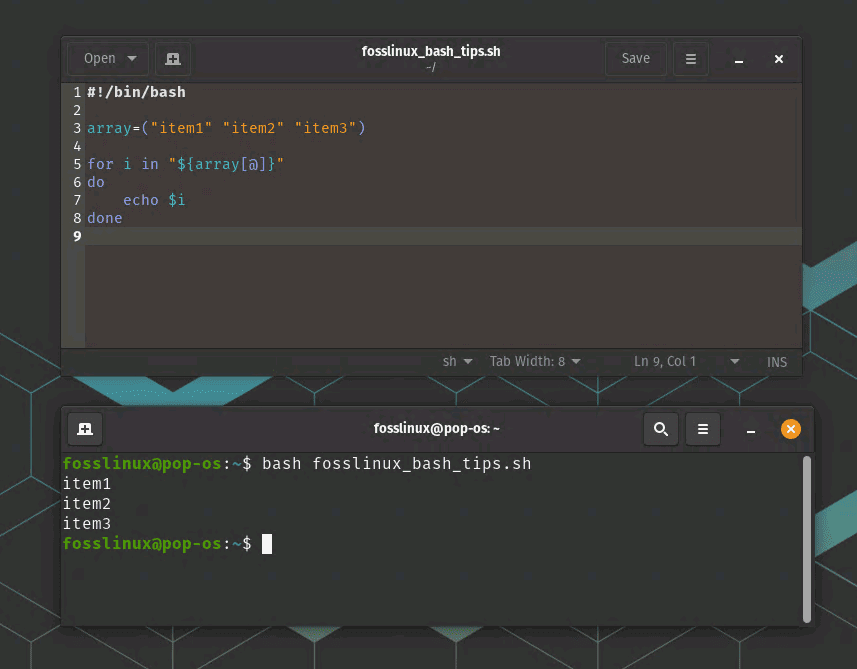
Loop to interate through items
While loops
While loops in Bash are used to execute a set of commands repeatedly until a specific condition is met. The basic syntax for a Bash while loop is while condition; do commands; done. The commands within the loop are executed as long as the condition is true. In Bash, condition can be a numeric or string comparison, a file test, or any other command that returns a true or false value. While loops are often used in Bash scripts to automate tasks that require continuous execution until a certain condition is met, such as monitoring a log file or waiting for a specific event. By using While loops, you can write more efficient and effective scripts that can save you time and effort in your daily tasks.
The basic syntax of the while loop is as follows:
while condition do statement done
In this example, the script checks condition and executes statement repeatedly until condition is false.
Here’s an example that prints the numbers from 1 to 5 using a while loop:
#!/bin/bash i=1 while [ $i -le 5 ] do echo $i i=$((i+1)) done
In this example, the script initializes i to 1, checks if i is less than or equal to 5 using the -le option, prints i, and increments i by 1 using the $((i+1)) syntax. This process is repeated until i is greater than 5.
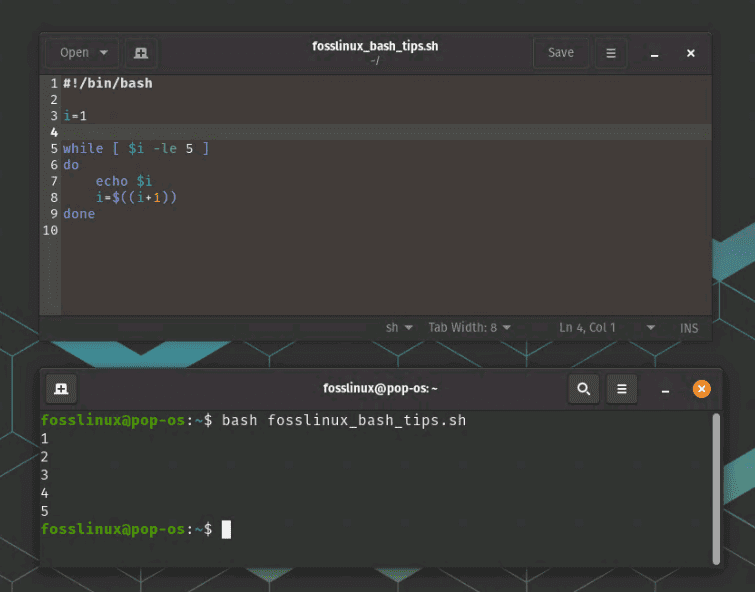
While Loop Usage
You can also use the while loop to read lines from a file:
#!/bin/bash while read line do echo $line done < /home/fosslinux/Documents/myparameters.txt
In this example, the script reads each line from the myparameters.txt file using the read command and assigns it to the line variable. It then prints each line using the echo command.
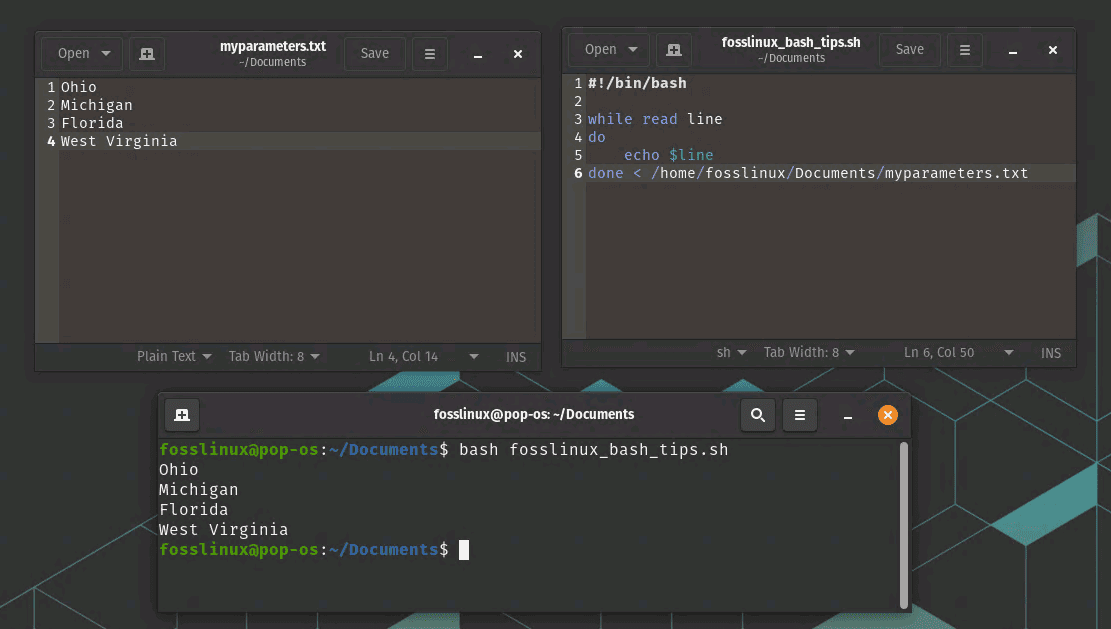
Read and print lines using while loop
Other helpful tips for writing great bash scripts using control structures
- Plan ahead: Before writing your Bash script, plan out the control structures you will need to accomplish your task. This will help you organize your code and make it easier to read and debug.
- Use the right control structure for the task: Choose the appropriate control structure based on the task you want to automate. For example, use If-then-else statements for conditional branching, For loops for iterating through a list of items, and While loops for executing a set of commands repeatedly until a condition is met.
- Keep your code organized: Use indentation and comments to make your code more readable and easier to understand. This will also make it easier to troubleshoot any issues that may arise.
- Minimize resource usage: Avoid using resource-intensive commands or operations within your control structures, as this can slow down the execution of your script. Instead, use efficient and lightweight commands whenever possible.
- Test your code: Always test your code thoroughly to make sure it works as expected. Use debugging tools like echo statements or log files to help you troubleshoot any issues that may arise.
Conclusion
Bash control structures such as if-then-else, for, and while loops are powerful tools that allow you to write scripts that perform complex operations and automate tasks. By using these control structures, you can perform different actions based on conditions, iterate through arrays or lists, and perform operations until a specific condition is met.
In this article, we discussed the basic syntax of the if-then-else, for, and while loops and provided practical examples to illustrate their usage. By mastering these control structures, you can write efficient and effective Bash scripts that can save you time and effort.
2 comments
Thanks
I am happy to be on this website