If you’re new to Linux systems, terms such as “Shell scripting” or “Bash scripting” may sound unfamiliar to you. However, Bash scripting is a useful skill to acquire as it can help you save time executing and performing various system tasks. Learning Bash scripting can also help you better understand the Linux command-line (Terminal) and automate multiple system tasks. This can make your work much more efficient and productive.
What is Bash?
Bash is a widely-used command language interpreter and Unix shell that is frequently utilized in the GNU operating system. The acronym “Bash” stands for “Bourne-Again-Shell,” which is a nod to its predecessor, the Bourne shell (sh). Bash has been the default shell for most Linux distributions and Apple macOS releases prior to macOS Catalina. It is also available on Windows 10 through the Windows subsystem for Linux.
When users want to perform various tasks using Bash, they typically do so within a text window known as the Terminal. They can type commands directly into the Terminal, which Bash then interprets and executes. Additionally, Bash is capable of executing commands written in a file, which is known as “bash scripting.” This feature enables users to automate repetitive tasks, execute complex commands with a single command, and more.
Difference between Bash scripting and Shell Scripting
It’s important to note that while Shell scripting and Bash scripting are similar, they are not exactly the same. Bash scripting involves the explicit use of the Bash shell, which is a widely used shell in Linux and Unix environments. However, Shell scripting is a more general term that encompasses other shells in addition to Bash. Some of the most commonly used shells include Korn (ksh), C shell (csh), Z shell (zsh), and Bash shell (sh).
It’s worth noting that while Bash is a powerful and flexible shell, it’s not the only option available. Each shell has its own unique features, syntax, and capabilities, and the choice of which one to use depends on the task at hand. However, it’s not uncommon for people to use the terms Bash scripting and Shell scripting interchangeably, even though they technically refer to different things.
If you’re interested in learning more about the different shells available for Linux, be sure to check out our post on the top 6 open source shells. It provides a detailed description of each shell, along with their pros and cons, so you can choose the one that best suits your needs.
In this post, we will explore Bash scripting which is a commonly used shell in many Linux distributions. To confirm the shell you are currently using on your Linux distribution, simply execute the command on the Terminal.
echo $SHELL

shell
From the image, you can see that my default interpreter is bash. I am currently using Ubuntu 20.04 LTS.
Bash Scripting Tutorial for Beginners
Bash scripts are essentially text files that contain commands written in the bash language. They are used to perform various tasks. To create these scripts, you only need a simple text editor such as gedit, leaf pad, vim, nano, and many others. It’s important to remember to save your scripts with a .sh extension and as plain text, rather than rich text. One of my preferred editors for writing scripts is the nano editor.
Naming, Assigning permissions, and Executing a Bash script
As mentioned earlier, it is important to use the .sh extension for your bash scripts. For instance, you can create a script with the name “myscript.sh”. However, you should be careful when naming your scripts. A common mistake is to save scripts as “test”. This may cause issues since there is a Unix built-in command named “test” that checks file types and compares values. To avoid any conflicts, you can use the “which” command to check if there is already a program with the same name as your script.
which [command] e.g which test

Which command
From the output above, we can see that test is already an inbuilt command. Therefore, to avoid any conflicts between programs, I will not name any of my scripts as “test.”
To make the script executable for every user, use the command below:
chmod +x [script.sh]
To make the script executable for the current user, use the command below:
chmod u+x [script.sh]
Now to run the script, execute the command below:
./[script_name]
With the above method, you will realize that you will need to use the cd
command to move into the directory containing the script or pass the path to the script. However, there is a workaround to this. Save your scripts in the bin
directory present in the home directory.
With recent updates, most distributions don’t come with the bin
folder by default. You will, therefore, be required to create it manually. By placing your scripts in this folder, you can execute the script by typing its name on the Terminal without using the cd
and ./
commands.
Bash Scripting guidelines
Before writing your first script, there are some guidelines that you need to understand when writing scripts.
- All your scripts should start with the line “#!/bin/bash.”
- Put every command on a new line.
- Every comment starts with a
#
symbol. - Commands are enclosed with
()
brackets.
The #!/bin/bash
(Hash-Bang Hack) statement is the path to the shell we will be using. If you are using another shell, then you would need to edit this path. We understand that all comments start with the #
symbol. However, when followed with a (!)
mark in the Hash-Bang Hack, the script is forced to execute with the shell pointed to by this line. In our case, it’s the Bash shell.
Your First Bash Script
Like most programming languages, we will write a script that prints the “Hello World” statement when executed. Open your favorite text editor and paste the commands below. Save the text file as fossHelloWorld.sh
or any other name that isn’t a built-in command.
#!/bin/bash #This script will print 'Hello World' on the Terminal echo "Hello World"
Once you have saved the file, make it executable by executing the command below:
chmod +x fossHelloWorld.sh
Now, run it with the command below:
./fossHelloWorld.sh

First script
The script above has only three lines. The first one is the Hash-Bang Hack, which we have discussed above, the second is a comment, and the third is the command that will be executed. Note, comments are never executed.
Let’s look at other bash scripting features that you can utilize.
Echo command
The Echo command is used to print output on the Linux command-line or Terminal. If you are a developer and have worked with PHP before, you must have come across this command too. Let’s write a small bash script to demonstrate how this command works.
#!/bin/bash #Let's print the first line echo "This is Fosslinux.com - With a new line" #Let's print the Second statement without a new line echo -n "This is Fosslinux - No new Line"
Save your script, assign the required permissions, and execute it.

Bash Echo
To understand the code, the first echo command prints a statement and goes to the next line. The second echo command has the -n
argument that prevents the script from going to the next line. You can use many other options with the Echo command, including variables, as we will see below.
Variables
Variables are a common and necessary part of any programming language. One can declare Bash variables in several ways. When assigning a value to any variable, you don’t need to use any symbols. However, when calling the variable’s value, you will need to use the $
sign at the variable’s beginning.
You can use variables on the Terminal or as scripts. Execute the commands below on your Terminal.
myWebsite = "Fosslinux.com" echo $myWebsite

Using Variables on terminal
Now let’s create a bash script that takes two inputs from the user and stores them in variables $x
and $y
. The script will then check whether the two values are equal or not.
#!/bin/bash echo "Enter the first number" read a echo "Enter the second number" read b if [[ $a -eq $b ]] then echo "Numbers are equal" else echo "Numbers are not equal" fi
Save the script and run it. From the image below, you can see we entered different values, and the script was able to check whether they are equal or not.
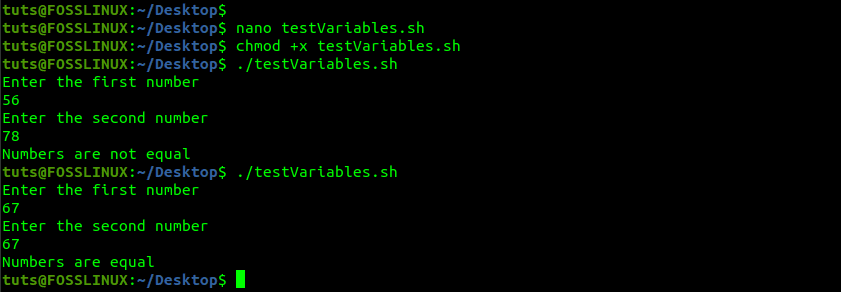
Bash variables
Now, let’s take a closer look at the code. The first two echo statement prompt the user to enter input the First and second numbers. The read
command will wait for the user to enter a number and store it to the specified variable. In our case, that’s a
and b
. We then have an if statement that checks whether the two values are equal or not.
Conditional statement
In any programming language, conditional statements control the flow of a program. A piece of code will execute only when a particular condition is met. Some of the most common conditional statements include “if-then-else” and “case.” Below is the basic syntax of the if-then-else statement.
if [[ condition ]] then <execute an instruction> else <execute another instruction/command> fi
Take a look at the script below, which makes use of the “if-then-else” conditional statement.
#!/bin/bash echo "Enter Your Age" read age if ((age > 18 )) then echo "You can go to the party" else echo "You are not eligible to go to the party" fi
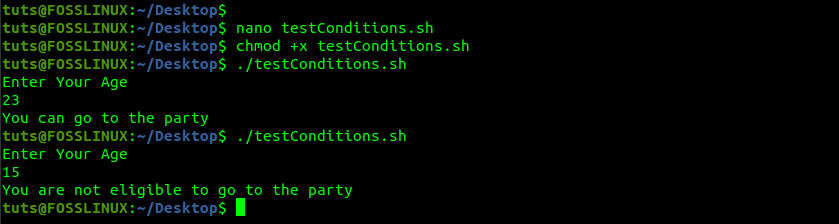
Bash conditional statements
Let’s go through the script above. We have the echo
statement that prompts users to enter their age number. The read
statement stores the age entered to a variable “age.” We then have an if-then-else conditional statement that checks whether the age is greater than 18 before instructing the user.
Loops
Loops are commonly used when you want to execute a piece of code multiple times. For example, if I wanted to echo 1000 lines of the statement “Welcome to Fosslinux,” I would require 1000 echo statements. However, with loops, all I need to do is specify the number of times I want the statement to be echoed or printed on the Terminal. Bash supports three types of loops; For Loop, While Loop, and the Until Loop. Let’s look at each of them.
The For Loop
A For Loop is used to iterate through a list of specified data or an array. Below is the basic syntax of the Bash For Loop;
for Value in 1 2 3 4 5 .. N do instruction1 instruction2 instruction-N done
or,
for VALUE in file1 file2 file3 do instruction1 on $VALUE instruction2 instruction-N done
Take a look at the script below. It iterates through the given days of the week and prints them on the Terminal line by line.
#Read a weekday name in each iteration of the loop for day in Monday Tuesday Wednesday Thursday Friday Saturday Sunday do echo "$day" done
Let’s save the script as ForLoop.sh
and execute it. Below is a screenshot of the output.
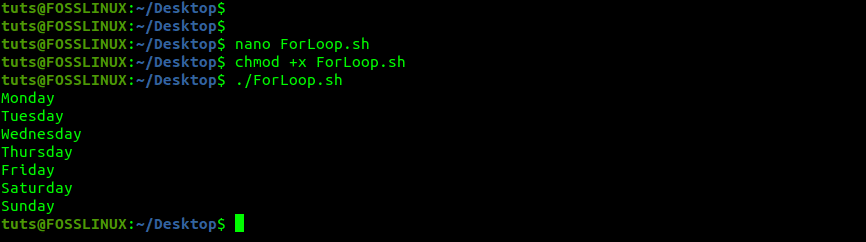
For Loop
That’s but a simple example of what you can do with the For Loop. You can also combine it with conditional statements to complete a particular task.
The While Loop
The While Loop executes a specific task while a particular condition remains True. In every iteration, the loop will first check the condition before executing the instructions. When the condition is False, the loop will terminate. The basic syntax of the bash While loop is as follows:
while [CONDITION] do [COMMANDS] done
For example, we can have a script that will print the statement “Welcome to FOSSLINUX.COM” 20 times until a variable X is set to 20. Let’s try and implement that practically. Take a look at the script below.
#!/bin/bash x=1 while [ $x -le 20 ] do echo "Welcome to FOSSLINUX.COM" x=$(( $x + 1 )) done
Let’s save the script as WhileLoop.sh
and execute it. Below is a screenshot of the output.
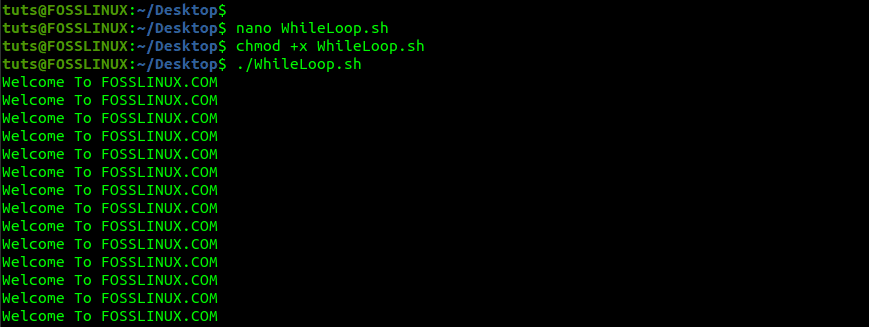
While Loop
Let’s take a look at the While Loop script above. We first declare a variable X giving it the value 1. We then create a While statement that outputs the statement “Welcome to FOSSLINUX.COM” while X is less than 20. Lastly, we increment X by one after every iteration.
The Until Loop
The Bash Until loops is used to execute a set of instructions or commands as long as the set condition evaluates to false. Below is the basic syntax of the Until Loop.
until [CONDITION] do [INSTARUCTIONS/COMMANDS] done
Similar to the While Loop, the Until Loop first evaluates the set condition before executing the instructions or commands. If the condition evaluates to false, instructions are executed. The loop is terminated when the condition evaluates to true. Take a look at the example below.
#!/bin/bash count=0 until [ $count -gt 5 ] do echo Counter: $count ((count++)) done
After we save and run the script above, we get the output below:
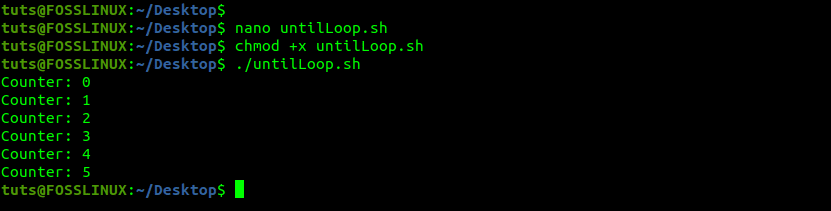
Until Loop
The scripts above first create a variable count and set it to zero(0). That is the condition that controls the flow of our program. The Until Loop checks whether the count value is greater than 5. If it is not, it will print the instructions passed. However, if the value is greater than 5, the loop will terminate immediately. Lastly, we increment the count value by 1 (count++).
Bash Functions
In programming and scripting, Functions are used to hold a reusable piece of code needed to perform a particular task. Therefore, if you have similar commands that you want to execute at different parts in your script, you don’t need to repeatedly write these commands. Enclose them in a function and execute the function instead.
Below is the basic syntax of the Bash function.
function_name () { <instructions/commands> }
or,
function function_name { <instructions/commands> }
Let’s create a simple script that makes use of functions. We first create a function called “myFunction,” which outputs the statement “Hello I am the first function” when called. The second function, “myCities,” is a little bit advanced as it takes arguments passed. The arguments are given after calling the function name. They are accessible in the function as $1.
#!/bin/bash myFunction () { echo "Hello I am the first function" echo "Hello I am the first function" echo } echo "This is the second function" function myCities () { echo Hello $1 } myFunction myCities Washington,D.C myCities Phoenix
After saving and executing the script, we get the output below.
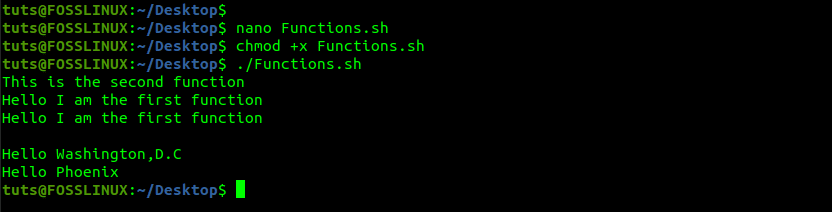
Functions
Bash Menus
When writing a script to perform an advanced task, you might need the user to select a single option from several options displayed on the Terminal. To achieve that, we will use the bash select
command.
It creates a simple menu script that will prompt the users to select their favorite Linux distributions from a list. Take a look at the script below.
#!/bin/bash #Print message for the user echo "Select your favorite Linux Distribution" select distro in Ubuntu Fedora OpenSUSE DeepIN Parrot ArchLinux Kali do #Print the selected value if [[ $distro == "Exit" ]] then exit 0 else echo "Selected Linux distribution is $distro" fi done
Let’s save the script as menu.sh and execute it. We get the output below.
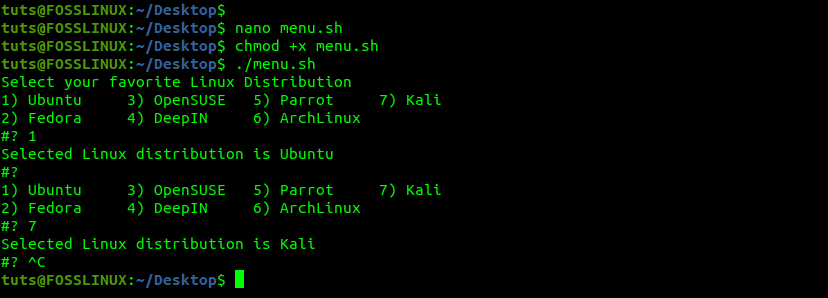
Bash Menus
In the script above, we have a list of Seven Linux distribution from the user will select one. After they make a selection, it is stored in the variable distro
and later printed by concatenating it with the string “Selected Linux distribution is.” Bash select menus can be quite useful, especially when you have multiple scripts performing different tasks, and one has to select the one they wish to execute.
Arithmetic
Every programming language supports the performing of arithmetic operations, and bash scripting is no exception. However, how we carry out arithmetic operations is a little different. Below is one of the ways you can perform arithmetic operations in bash. We will look at four types of arithmetic operations – Sum, division, and multiplication.
#!/bin/bash # Calculate the sum result=$((70+15)) # Print sum value echo "sum = $result" # Calculate the division result=$((100/25)) # Print division value echo "division = $result" #Calculate Multiplication result=$((15*15)) echo "Multiplication = $result"
Let’s save the above script as arithmetic.sh and execute it. We get the output below.

Arithmetic
Conclusion
If you are new to Bash scripting, then you might find it a bit challenging to get started. However, there is no need to worry, as here is a complete beginner’s guide to help you get started with Bash scripting. Keep in mind that this guide covers only the basics, and there is much more to learn about Bash scripting. If you want to advance your skills, then I would recommend checking out the GNU’s Bash Reference Manual. It provides advanced information on Bash scripting and can help you become an expert in no time. If you face any difficulties or have any questions related to this post, then please feel free to leave a comment below.
1 comment
Since the vast majority of Linux distros use the Bash shell, Bash scripting == Shell scripting. I have not seen any articles or manuals or guides to Zsh Scripting, Csh Scripting or Fish Scripting.