When developing Shell scripts, you may come into situations where you must execute an action depending on whether a file exists. The test command in bash may be used to discover whether a file exists and its type.
In Linux, there are various ways to check a file’s availability. The “test” command in bash scripting is among the most essential methods for verifying the presence of a file.
If a Linux Bash script depends on the presence of specific files or directories, it cannot simply assume that they exist. It must ensure that they are indeed present. Here’s how to go about it.
1. Make No Assumptions
When developing a script, you can’t make assumptions about what’s present and what isn’t on a computer. This is especially true if the script is distributed and run on various systems. The script will eventually run on a computer that does not satisfy your assumptions, and it will fail or execute unpredictably.
Everything we value or produce on a computer is saved in a file. After that, the files are kept in a directory. Scripts may read, write, rename, delete, and move files and directories like the command line.
As a human, you have the benefit of examining the contents of a directory and determining whether a file exists or whether the anticipated directory even exists. When a script makes a mistake when modifying files, it might have significant and harmful consequences.
Bash has a comprehensive collection of tests for detecting files and directories, as well as testing for many of their characteristics. It is simple to include them in scripts, but the advantages in terms of sturdiness and fine control are significant.
2. Primary Expressions
We can quickly detect if a file exists, is executable or readable, and much more by integrating the if statement with the relevant test from a vast array of file and directory tests. Below are some helpful test syntax statements:
- -b: Gives a “true” response if the file in question is a “block special file.”
- -c: Gives a return value of true if the file contains special characters.
- -d: Determines whether the “file” is a directory.
- -e: Gives a return value of true if the file already exists.
- -f: Gives a true response if the file in question exists and is of the ordinary file type.
- -g: Determines whether or not the file has the setgid permission set (chmod g+) and returns true if it does.
- -h: Gives a “true” response if the file in question is a symbolic link.
- -L: Gives a “true” response if the file in question is a symbolic link.
- -k: gives a return value of true if the file in question has the sticky bit enabled (chmod +t).
- -p: Gives a “true” response if the file in question is a named pipe.
- -r: Gives a return value of true if the file can be read.
- -s: Gives a return value of true if the file in question does not exist or if it contains data.
- -S: Gives a “true” response if the file in question is a socket.
- -t: Gives the value true if the file descriptor is accessed from a terminal.
- -u: Determines whether or not the file has the setuid permission set (chmod u+) and returns true if it does.
- -w: Determines whether or not the file can be written to and returns true if it can.
- -x: Gives a return value of true if the file can be executed.
- -O: If you own the this function returns true.
- -G: Gives a “true” value if your group owns the resource.
- -N: Is a switch that, when used, tells the program whether or not the file has been altered since the previous time it was read.
- !: represents the NOT operator in logic.
- &&: is the “AND” operator in logical expressions.
- || is the logical operator for OR.
- The -e test has superseded the -a test; Thus, the list begins with -b. This is because the -a test is now considered obsolete.
The purpose of this guide is to converse about the presence of a file on your system using bash scripting: With all that said, let us dive deep and learn how to check if a file exists in bash.
Checking if a file exists in bash
This blog article will go through distinct techniques to verify if a file exists in bash. This is a crucial ability to know when working with files in a Linux environment. Each approach has advantages and disadvantages, so it is critical to grasp them before picking which one to apply. Let’s get this party started!
How to use bash scripting to check for file existence
The methods highlighted herein will be of much importance to check for file existence using bash scripting:
Check if a file exists
There are various methods to check if a file exists. Try out any technique highlighted in this article tutorial guide:
Method 1: By inputting the file name into the terminal:
To begin, run the following command to create a bash script file:
touch fosslinux.sh
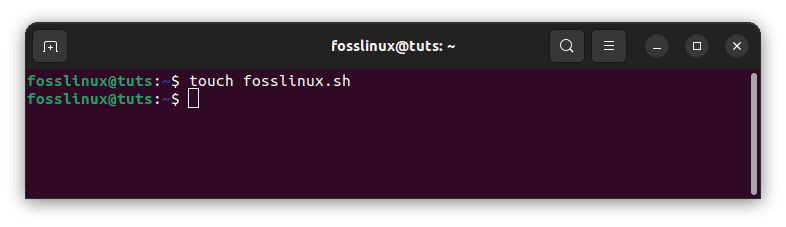
create fosslinux script
The file I produced is called “fosslinux.sh,” and the “.sh” extension denotes a shell script file.
To open a bash script run the following command and remember to replace name with your actual file name:
nano fosslinux.sh
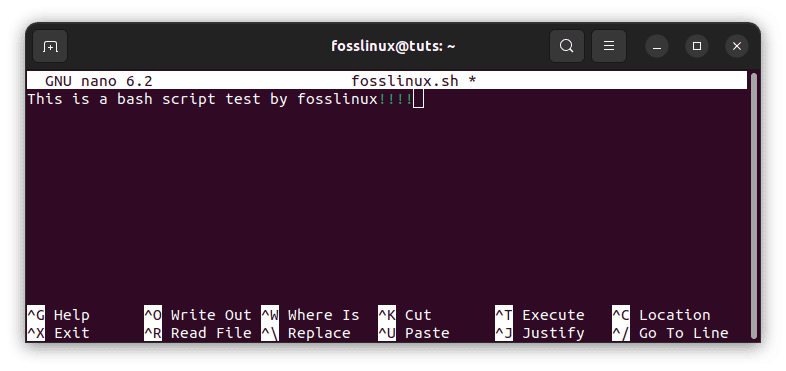
edit script
In any text editor, open “fosslinux.sh.” Then compose the script and save it by clicking “save.”
One approach to finding a file is to ask the user for a filename in the terminal.
To verify the presence of a file, use “-f.”
Write the following script:
#!/bin/bash echo "Enter your filename." read fosslinux1 if [ -f "$fosslinux1" ] then echo "File exists." else echo "File does not exist" fi
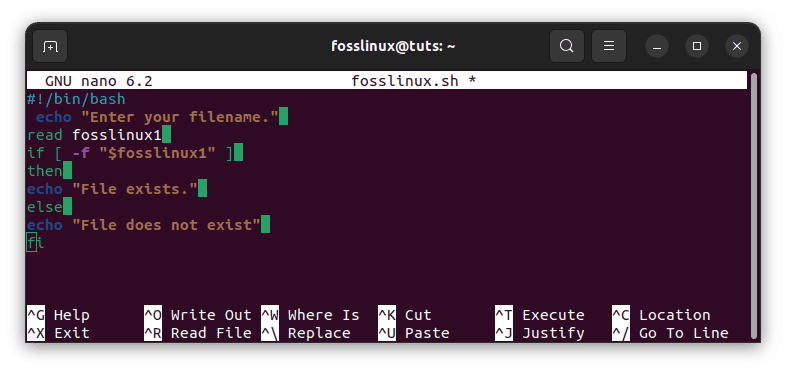
edit bash script
Return to the console and execute the file to see the output:
./fosslinux.sh
Note: Ensure you replace ‘filename’ with your actual filename.
Once you execute the code above, you will encounter a permission denied message on your console.
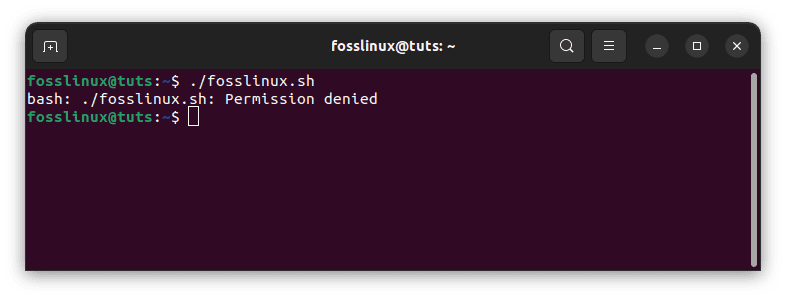
permission denied
Don’t worry. Proceed and make the file executable by running the line of code below. But remember always to replace your filename with the file’s actual name.
chmod +x fosslinux.sh
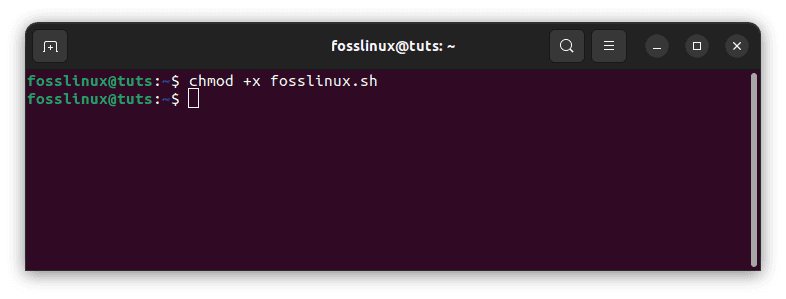
make file executable
Simply input the name of the file, and it will print the results:
Method 2: By entering the file name when composing the script:
Another method for finding a file is to provide the file’s name while the script is being written. There are three different ways for us to check whether or not the file is available. The first one uses the “test” command, the second one uses “if” with an expression enclosed in square brackets, and the third one also uses “if,” but this time it uses double square brackets as seen in the following example:
- “test EXPRESSION.”
- “if [EXPRESSION]”
- “if [[EXPRESSION]]”
Let’s get a better grasp on it with several examples:
1. test [Expression]
Simply cut and paste the provided script into the editor, then save the file:
#!/bin/bash filename=foss1 if test -f "$filename"; then echo $"file exists." else echo $"file does not exist" fi
![test [expression]](https://b1490832.smushcdn.com/1490832/wp-content/uploads/2022/08/test-expression-1.png?lossy=2&strip=1&webp=1)
test [expression]
./fosslinux.sh

file does not exist
The code outputs the message “File does not exist” since there is no such file in my directory, which is why the message appears.
2. if [Expression]
To determine whether or not the file already exists, please copy and paste the following script:
#!/bin/bash filename=fosslinux.txt if [ -f "$filename" ]; then echo $"filename exists" else echo $"filename does not exist" fi
![if [expression]](https://b1490832.smushcdn.com/1490832/wp-content/uploads/2022/08/if-Expression.png?lossy=2&strip=1&webp=1)
if [Expression]
./fosslinux.sh
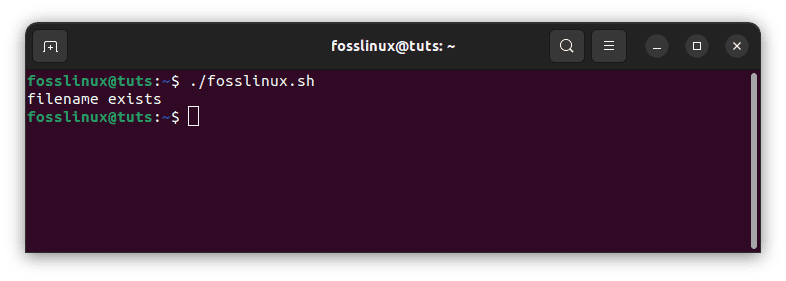
filename exists output
3. if [[Expression]]
Make a copy of the script that is provided below, and then paste it into the terminal:
#!/bin/bash filename=fosslinux if [[ -f "$filename" ]]; then echo $"filename exists" else echo $"filename does not exist" fi
![if [[expression]]](https://b1490832.smushcdn.com/1490832/wp-content/uploads/2022/08/if-Expression-1.png?lossy=2&strip=1&webp=1)
if [[Expression]]
./fosslinux.sh
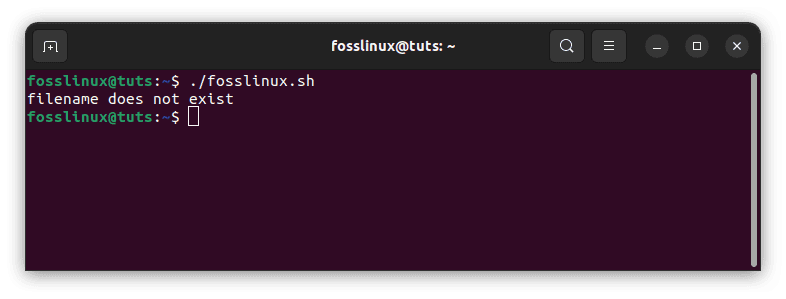
filename does not exist
Method 3: Check to see if a file exists using the bash test command
The first way we’ll go through is the test command. This is a Bash command that may be used to test various things. In this situation, we’d like to use it to see if a file exists. This command’s syntax is as follows:
test -e /path/to/file
This command will yield a 0 exit code if the file exists. A non-zero exit code will be yielded if the file doesn’t exist. So, we can use this command to see if a file exists as follows:
If test -e /path/to/file returns true, then echo “File exists.”
else echo “File does not exist”
We can accomplish this with a single command like this.
test -e /path/to/file || echo "FILE does not exist."
Example 1:
#!/bin/bash test -f fosslinux.txt && echo "file exists"
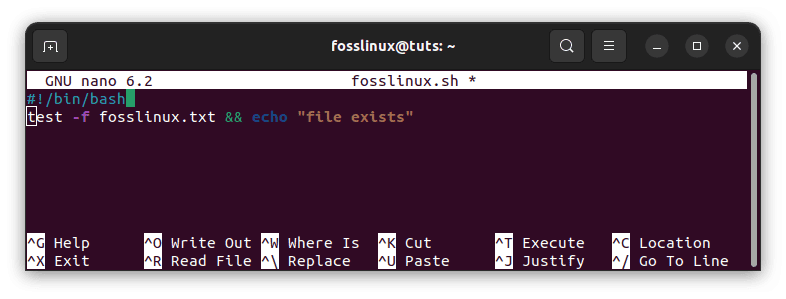
check if a file exists
Example 2:
#!/bin/bash [ -f fosslinux.txt ] && echo "$file exists"
Example 3:
#!/bin/bash [[ -f fosslinux.txt ]] && echo "$file exists"
Return to the console and execute the file to see the output:
./fosslinux.sh
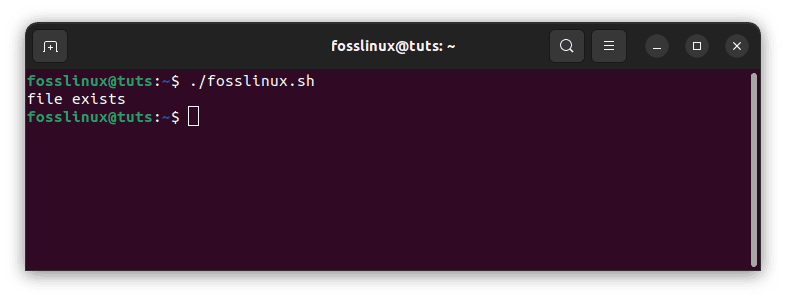
file exists output
Method 4: Check to see if a file exists using the bash if statement -e option
The if statement -e option is the best Linux command to verify if a file exists in bash. The -e option in bash is a built-in operator for checking file existence. This command will produce a 0 exit code if the file exists. A non-zero exit code will be returned if the file does not exist.
This operator’s syntax is as follows:
If [-e /path/to/file] is present, then echo "File exists." else echo "File does not exist"
We can accomplish this with a single command.
[ -e /path/to/file ] && echo “File exists.” || echo "File does not exist"
Example:
[ -e fosslinux.txt ] && echo “File exists.” || echo "File does not exist"
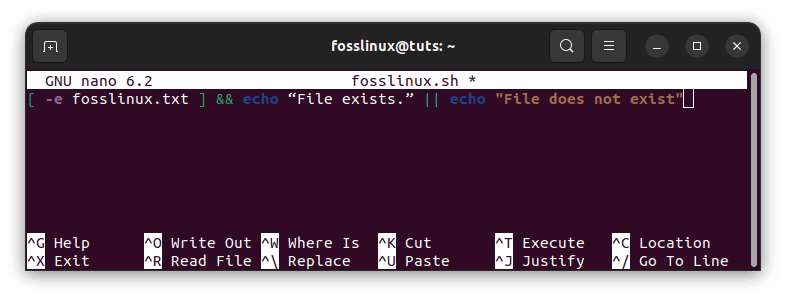
check if file exists -e option
Method 5: Check to see if a file exists using the -f flag in the bash if statement
The third technique will be to use the -f option in the if statement. The -e option determines if the file path exists, whereas the -f option determines whether the file path exists and whether it is a normal file. These operators have the following syntax:
If [-f /path/to/file] is present, then echo “File exists.”
else echo “File does not exist”
We can accomplish this with a single command line.
[ -f /path/to/file ] && echo “File exists.” || echo "File does not exist"
Example:
[ -f fosslinux.txt ] && echo “File exists.” || echo "File does not exist"
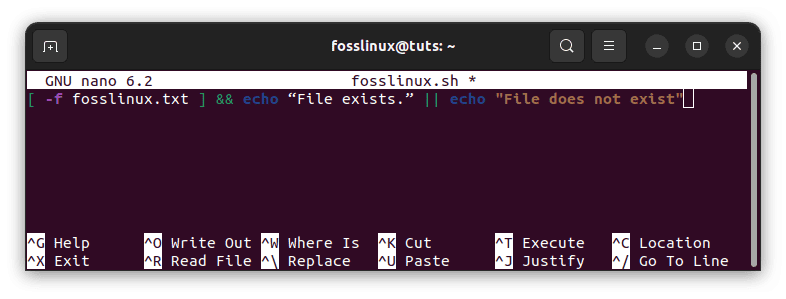
check if file exists -f flag
Check if a directory exists
There are two methods that we shall use to check if a directory exists using bash scripting:
Method 1: While writing a script, enter the directory name
To validate the presence of a directory, use the “-d” flag.
In the script below, “fossdir” is the variable in which you keep the file you are looking for; in my case, I want to see if the directory “fosslinuxDir” exists or not.
#!/bin/bash dir11=fosslinuxDir if [ -d "$fossdir" ] then echo $"Directory exists." else echo $"Directory does not exist" fi
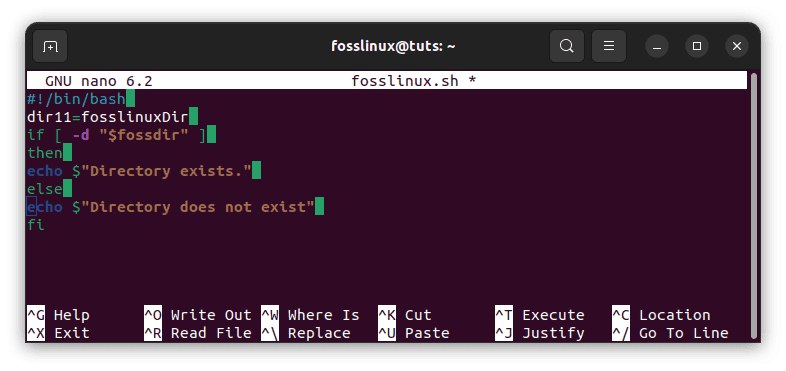
check if a directory exists
Create a new directory by executing this command:
mkdir Dir
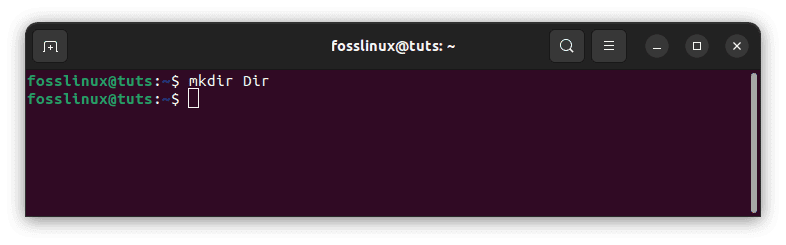
create new directory
Once done, run this line of code to output the results:
./fosslinux.sh
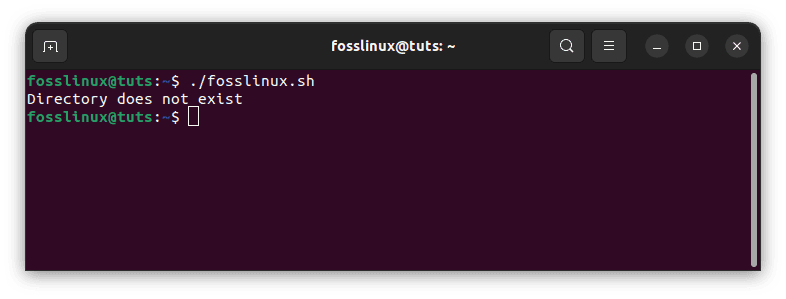
directory does not exist
Method 2: By typing the directory name into the terminal
When you execute the command in the terminal to see if the directory exists, you must specify the directory name that you are looking for:
#!/bin/bash echo "type your directory name." read fossDir if [ -d "fosslinuxDir" ] then echo $"directory exists." else echo $"directory does not exist" fi
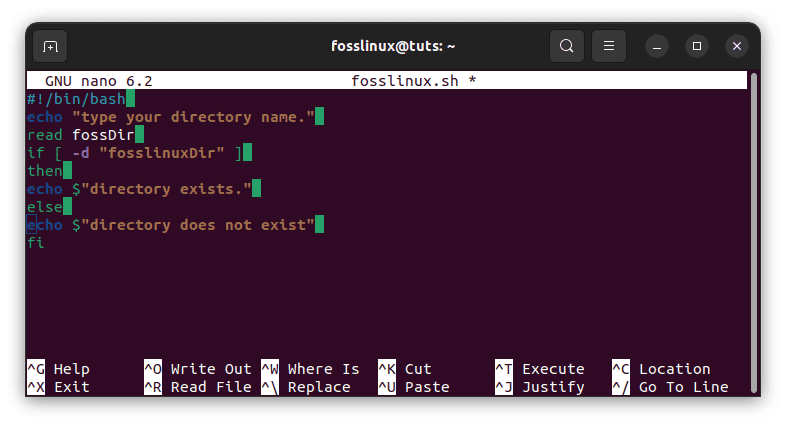
check if directory exists using name
Once you are done, execute the line of code provided herein to output the results:
./fosslinux.sh
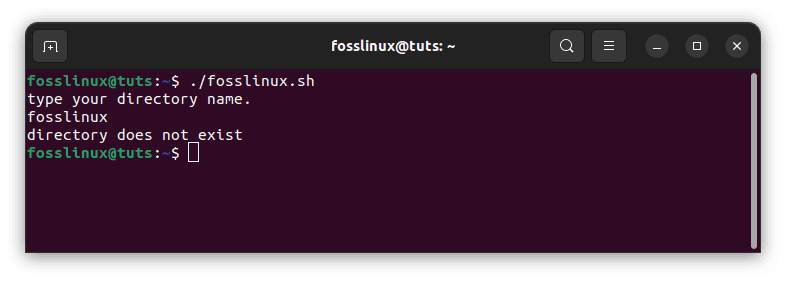
directory does not exist output
Method 3: Without using the “if” statement, check the directory existence
To determine whether a directory exists, use the following statements:
#!/bin/bash [[ -d fossDir ]] && echo "directory exists"
Output:
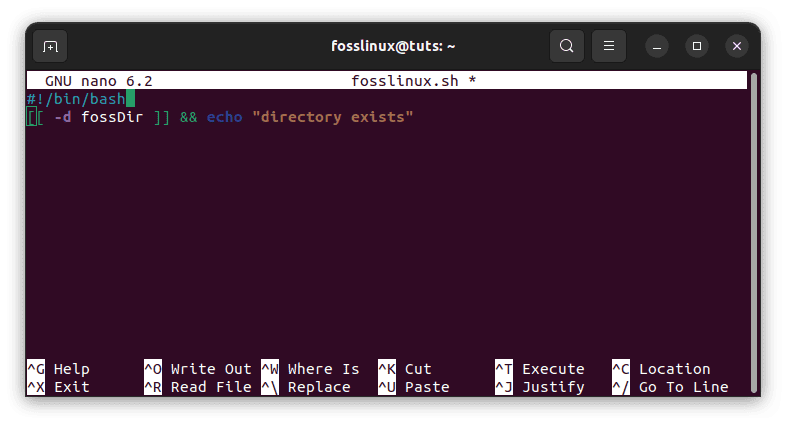
check if directory exists using double quotes
#!/bin/bash [ -d fossDir ] && echo "directory exists"
Output:
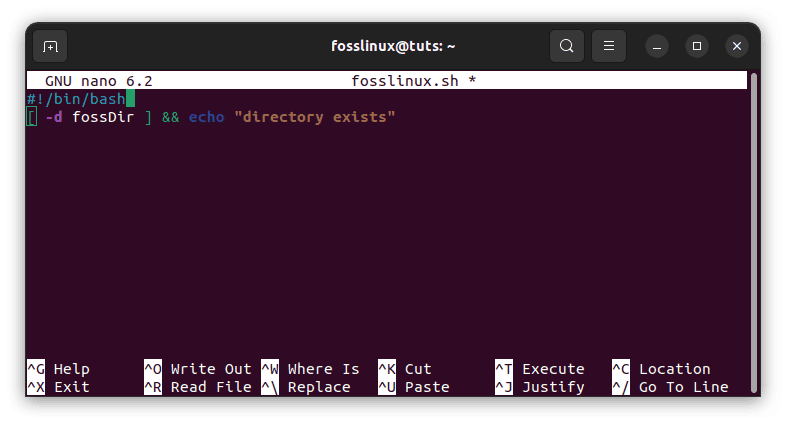
check if directory exists using single quote
Return to the console and execute the file to see the output:
./fosslinux.sh
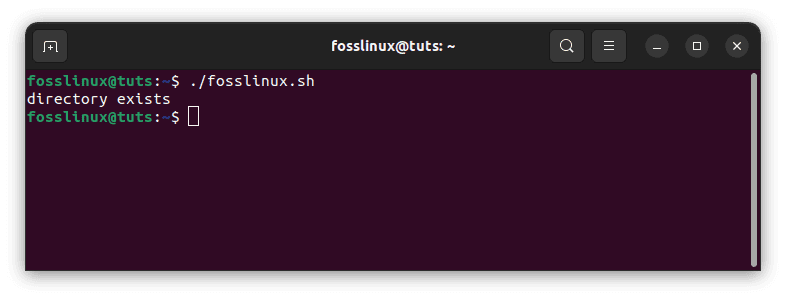
directory exists output
Multiple file/directory checks:
1) Using “if” statements to check several files:
Instead of using nested “if/else” statements, use the “-a” switch to verify the presence of several files:
#!/bin/bash if [ -f foss_linux.txt -a -f fosslinux.txt ]; then echo "Both files exist." fi
An alternative method is:
#!/bin/bash if [[ -f foss_linux.txt && -f fosslinux.txt ]]; then echo "Both files exist." fi
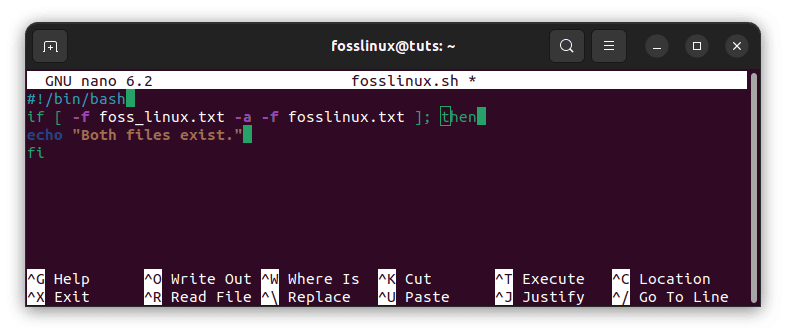
check multiple files
Return to the console and execute the file to see the output:
./fosslinux.sh
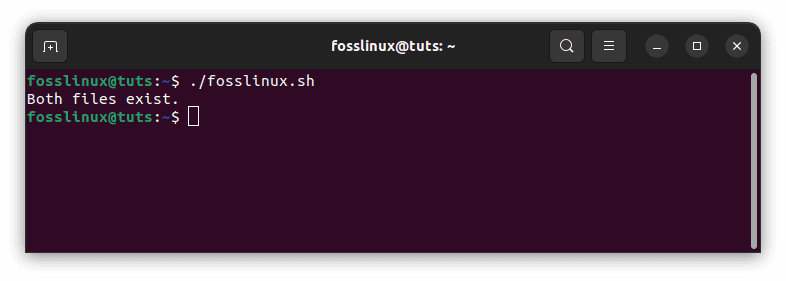
both files exist
2) Multiple file checks without the use of the “if” statement:
To examine many files at the same time without using “if,” use the following statement:
#!/bin/bash [[ -f foss_linux.txt && -f fosslinux.txt ]] && echo “Both files exits.”
Output:
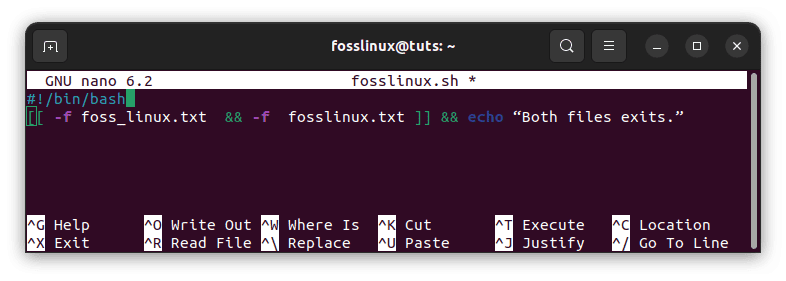
check multiple files using double quotes
#!/bin/bash [ -f foss_linux.txt && -f fosslinux.txt ] && echo “Both files exits.”
Output:
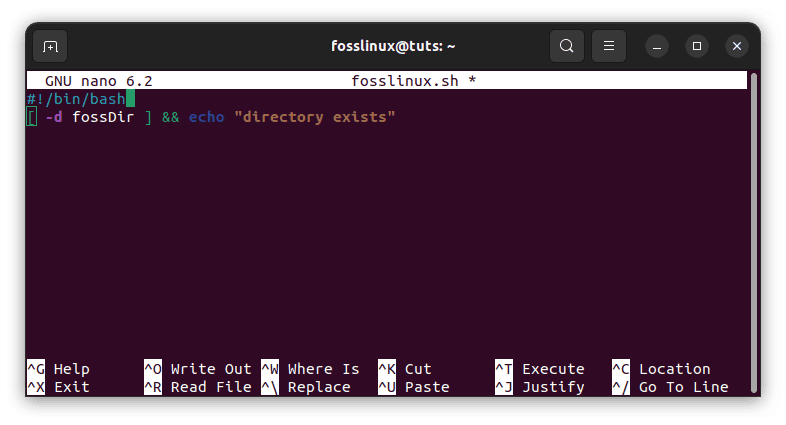
check if directory exists using single quote
Return to the console and execute the file to see the output:
./fosslinux.sh
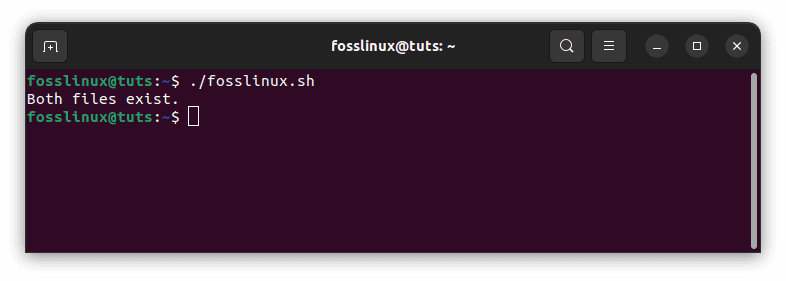
both files exist
Conclusion
The usage of bash scripting to verify a file or directory has been demonstrated throughout this article. When checking the availability of a file, we made use of a variety of different choices. Test and Don’t Assume. Assumptions will, sooner or later, lead to the occurrence of undesirable events. First, do the test, then respond as necessary. The more you know, the more authority you have. You may provide your scripts with the information they require by testing them.