Programming languages are built on a foundation of many core concepts, including loops. Loops come in handy when you need to execute a set of commands several times until a particular condition is met. Loops are a valuable tool for automating repetitive tasks and can be found in scripting languages such as Bash. The for loop, the while loop, and the until loop are the three fundamental iteration constructs in Bash scripting.
This guide will walk you through the fundamentals of using while loops in Bash. In addition, we will demonstrate how to change the course of a loop by utilizing the break and continue statements in the appropriate places.
In a Linux Bash script, the while loop ensures the script will continue to run so long as the condition that was programmed remains accurate. When you need to repetitively execute a set of commands a certain number of times, or when you desire to create an infinite loop, while loops are valuable tools to have at your disposal. To teach you how while loops in a Bash script are written and what kind of functions they perform, this tutorial will walk you through several example scripts that contain while loops.
Bash While Loop
The Bash While Loop is a statement used to execute a block of statements repeatedly based on the boolean result of articulation for as long as the expression evaluates to TRUE. This loop statement is used to execute a set of statements recurrently based on the boolean result of an expression.
Syntax
while [ expression ]; do statement(s) done
There can only be one condition in the expression. If the expression has multiple conditions, the while loop syntax is as follows:
while [[ expression ]]; do statement(s) done
The while loop one-liner syntax is as follows:
while [ condition ]; do commands; done while control-command; do Commands; done
The “while loop” statement has the following salient points:
- The condition is examined first, and then the commands are carried out.
- The ‘while’ loop is equally capable of carrying out all of the tasks that can be accomplished by the ‘for’ loop.
- As long as the statement conditions are met, the commands between the words “do” and “done” will be repeated.
- A boolean expression can be used in the place of an argument for a while loop.
How exactly does it operate?
One example of a restricted entry loop is the while loop. It indicates that the condition is evaluated before carrying out the commands contained within the while loop. In the event that the condition is found to be accurate, the set of commands that follow that condition will be carried out. If this condition is not satisfied, the loop will be broken. Therefore, control of the program will be passed on to the command that comes after the ‘done’ statement.
Bash While Loop Examples
In the bash script, the keywords do and done are used to define the beginning and end blocks of the while loop, respectively. The starting point of the loop is where the condition that will cause the loop to end is defined. Launch a text editor to compose a bash script and experiment with the while loop examples provided below.
Example 1: Fixed-number loop iterations
One of the applications of a while loop would be to iterate over the same section of a script a predetermined number of times. Controlling the number of times a script is run can be accomplished with the help of an incrementing variable. Take, for instance, the following script, an example of a straightforward countdown timer for five seconds.
#!/bin/bash i=5 while [ $i -gt 0 ] do echo Countdown expires in $i... ((i--)) sleep 1 done echo Countdown complete!
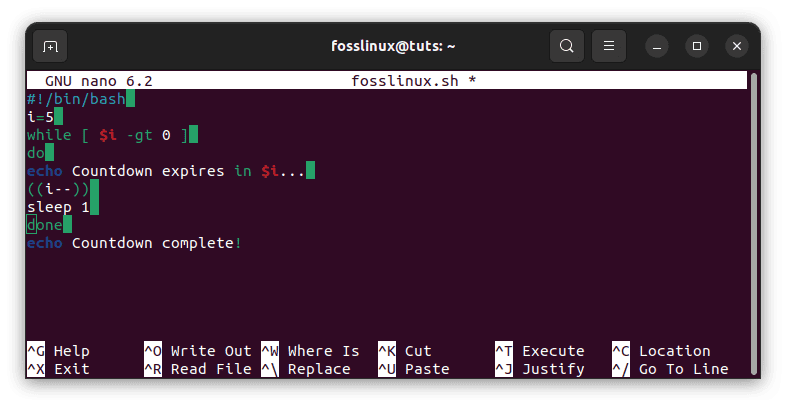
fixed loop iterations
The value 5 is allotted to the variable $i when it is first declared. The condition of the while loop will be considered met, and the loop will be carried out whenever the value of this variable is greater than 0. In this particular scenario, the loop will be executed five times before the value of the $i variable is decremented all the way down to 0. A countdown timer for five seconds will be created as a result of this action. When we run the script, the following is what appears on the screen of our terminal:
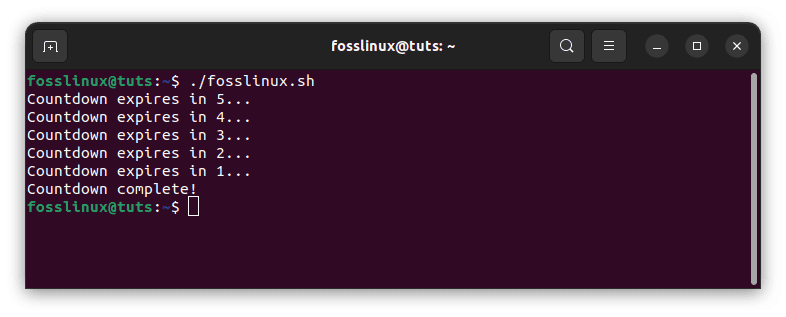
fixed loop iterations output
Example 2: Read a file using the while loop
If you intend to read a file line by line and then process what you’ve read, the while loop is the best choice. Use the following information in a brand-new text file named fosslinux.txt. Following line 2 is a blank line intentionally left there to demonstrate the behavior of how empty lines are dealt with when using loops.
14:00 FossLinux tutors arrive 14:30 FossLinux authors introduction 16:30 FossLinux band entertains the crew
Note: You can check the contents of the fosslinux.txt file by running the line of code provided herein:
cat fosslinux.txt
Together with an input redirection operator, which will send the file name to the while loop, we will use the read command to receive input from redirection and save it in a variable. Additionally, the while loop will be given the file name. The word split boundary will be determined by the read command by using the IFS that is set as the default.
while read line do echo $line done < fosslinux.txt
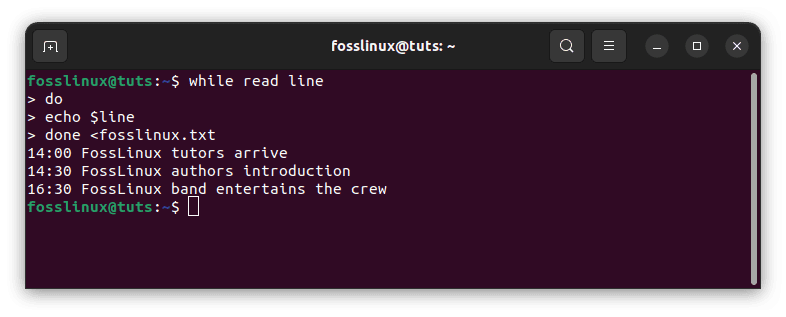
send filename to while loop
The file fosslinux.txt is redirected to the while loop in the example that was just presented. The read command then reads each line of the file, stores it in the variable “line,” and then continues to process it within the loop. When you use this method, empty lines are not skipped, which is a behavior that you probably do not want because it saves you time. Therefore, you will need to create blank lines so they can be skipped intentionally.
However, a few different approaches are available for removing empty lines from the input file. You can use sed, awk, conditional statements, and so on. Before I use a while loop to iterate over the lines, I like to clean them up by first running them through awk and then sed as illustrated herein:
# USING SED $ sed '/^[[:space:]]*$/d' fosslinux.txt 14:00 FossLinux tutors arrive 14:30 FossLinux authors introduction 16:30 FossLinux band entertains the crew # USING AWK $ awk 'NF' fosslinux.txt
Check the outputs to see how sed and awk were used respectively to do away with the spaces in question.
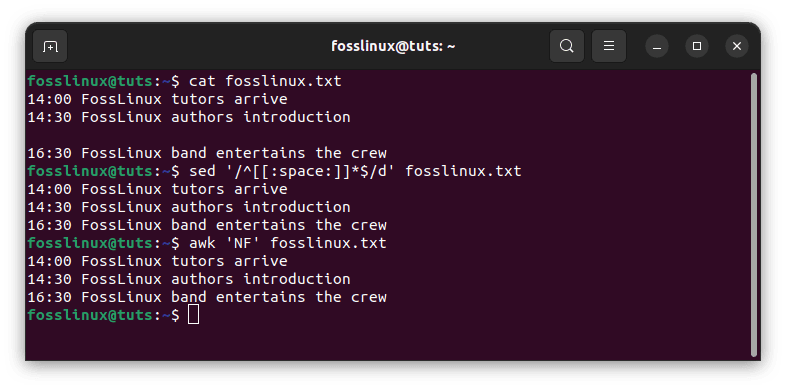
Remove extra space
Example 3: Using continue statement to omit certain step
The following line of code should be placed in a bash file that you have created and titled fosslinux.sh. The loop will iterate five times in this demonstration, but it will only output 7 of the 10 places each time. The continue statement will be invoked when the loop iterates for the third time, at which point the loop will go to the next iteration without printing the text in the third position.
#!/bin/bash # Initialize the counter n=0 # Iterate the loop 10 times while [ $n -le 10 ] do # Increase the value of n by 1 (( n++ )) # Ascertain the value of n if [ $n == 7 ] then continue fi # Print the undercurrent value of n echo "Position: $n" done
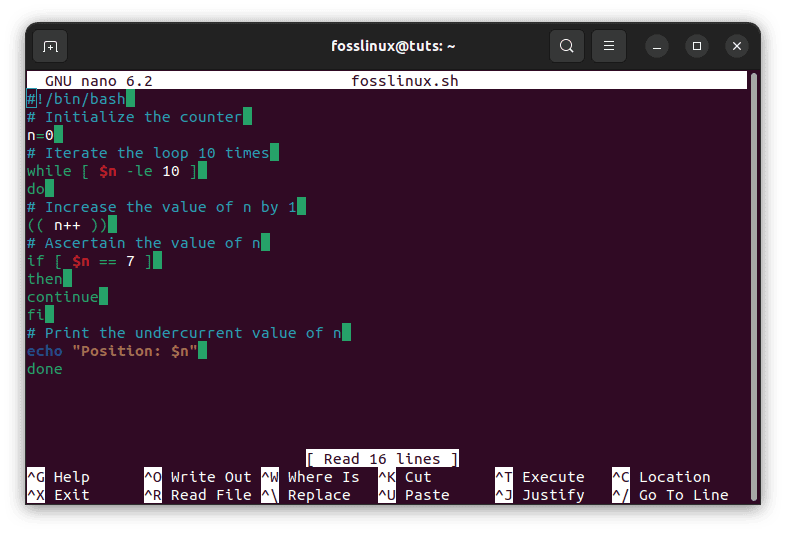
continue statement
Output:
When the aforementioned script is executed, the output shown below will appear:
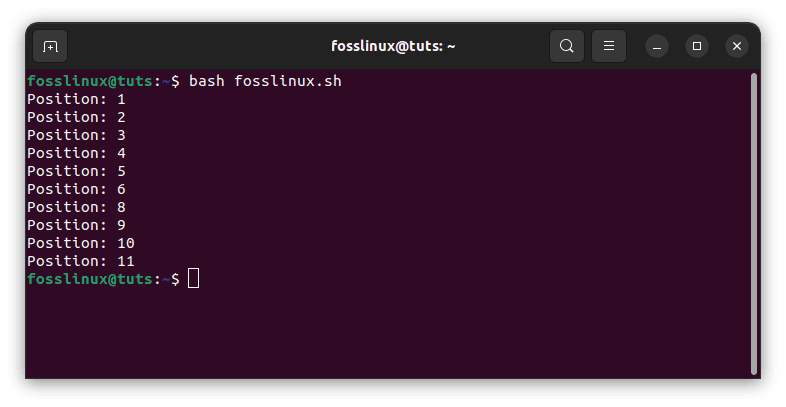
continue statement output
Example 4: Implementing a conditional exit with the break statement
A particular condition can be used with the break statement to cause the program to exit the loop at an earlier point. The following line of code should be placed in a bash file that you have created and titled fosslinux.sh. The loop is programmed to execute 10 times the defined iteration in this case. However, the iteration will end when the counter number reaches 6.
#!/bin/bash # Initialize the counter n=1 # Iterate the loop 10 times while [ $n -le 10 ] do # Ascertain the value of n if [ $n == 6 ] then echo "terminated" break fi # Print the undercurrent value of n echo "Position: $n" # Increase the value of n by 1 (( n++ )) done
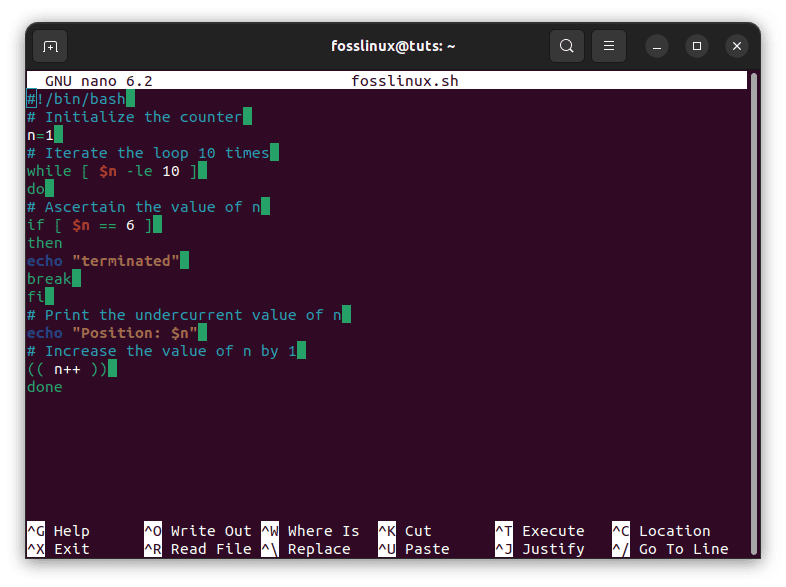
break statement
Output:
When the aforementioned script is executed, the output shown below will appear:
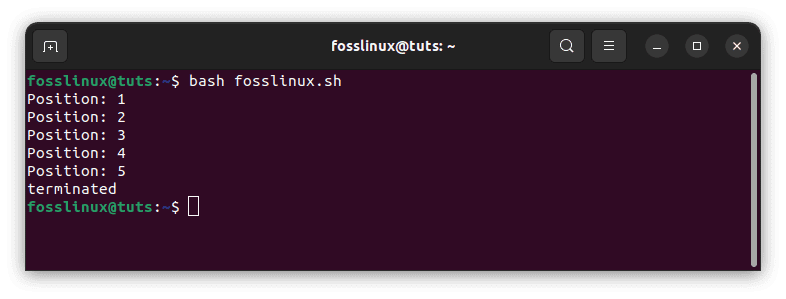
break statement output
Example 5: Iterate the loop for a predetermined number of times
Iterate the loop a predetermined number of times, as shown below.
Create the following code in a bash file and save it under fosslinux.sh. At this point, the loop will iterate five times, and after each iteration, it will output the current value of the counter.
#!/bin/bash # Initialize the counter n=1 # Iterate the loop 10 times while [ $n -le 10 ] do # Print n's value in each iteration echo "Executing $n time." # Increase the value of n by 1 (( n++ )) done
Output:
When the aforementioned script is executed, the output shown below will appear:
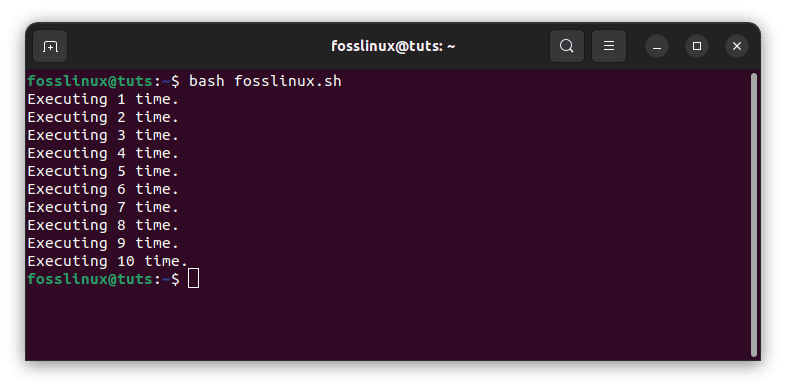
iterate loop severally output
Example 6: Read the argument from the command line with options
The following code should be placed in a bash file that you have created and titled fosslinux.sh. In this case, the loop reads the arguments and parameters from the command line. Immediately following the execution of the script, the formatted argument values will be printed out if the three-argument values are compatible with the valid option.
#!/bin/bash # Read the argument from the command line with the option using loop while getopts n:a:e: OPT do case "${OPT}" in n) name=${OPTARG};; a) address=${OPTARG};; e) email=${OPTARG};; *) echo "Invalid option." exit 1;; esac done # Print out the argument values printf "Title:$name\nAddress:$address\nEmail:$email\n"
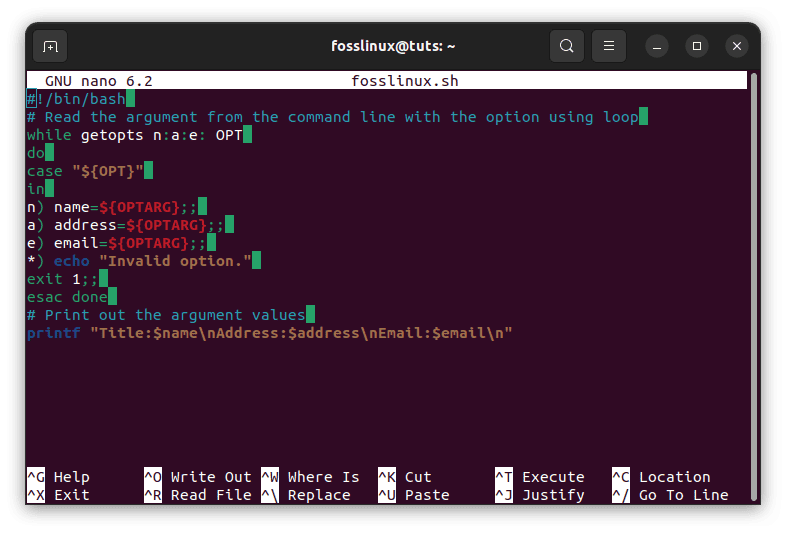
read arguments from commandline
Output:
When the aforementioned script is executed, the output shown below will appear:
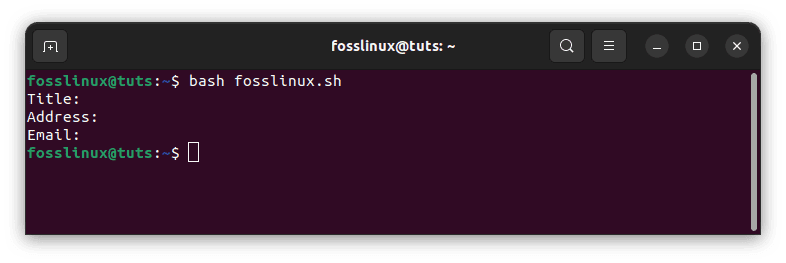
read arguments from command line output
Example 7: Write content into a file
Write the following statement in a bash file and save it under fosslinux.sh. In this instance, the user’s input will determine the file’s name into which the text content will be written. After inputting the contents of the file, the user is required to press the Ctrl and D keys simultaneously.
#! /bin/bash echo -n "Input the filename:" # Take the filename that will be created read filename # Read the file's content from the terminal while read line do echo $line >> $filename done
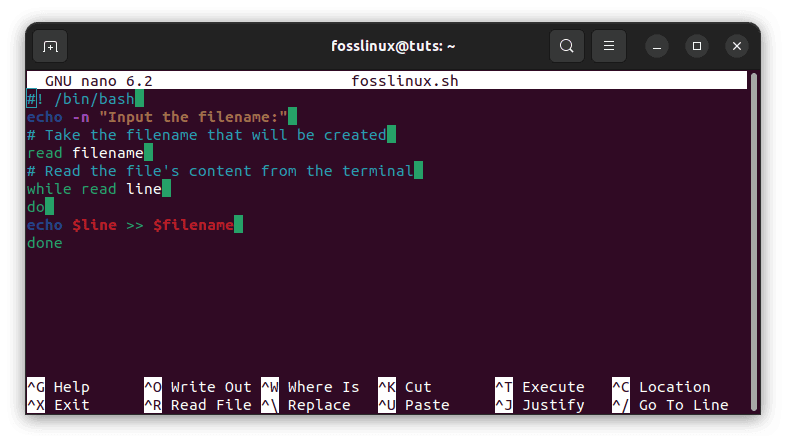
write content into a file
Output:
When the aforementioned script is executed, the output shown below will appear:
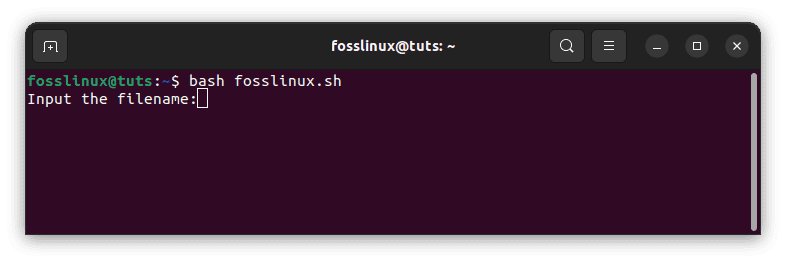
write content into a file output
Example 8: Read the file one line at a time
The following code should be placed in a bash file that you have created and labeled fosslinux.sh. At the time of the program’s execution, a filename will be supplied as the initial argument on the command line. In the event that the file does not exist, an error message will be displayed instead of the contents of the file, which will be printed using the loop if the file does exist.
#!/bin/bash # Ascertain whether the command-line argument value is given or not if [ $# -gt 0 ]; then # Allocate the filename from the command-line argument value filename=$1 # Read the file line by line while read line; do # Print each line echo $line done < $filename else # Print out the message if no argument is provided echo "The argument value is missing." fi
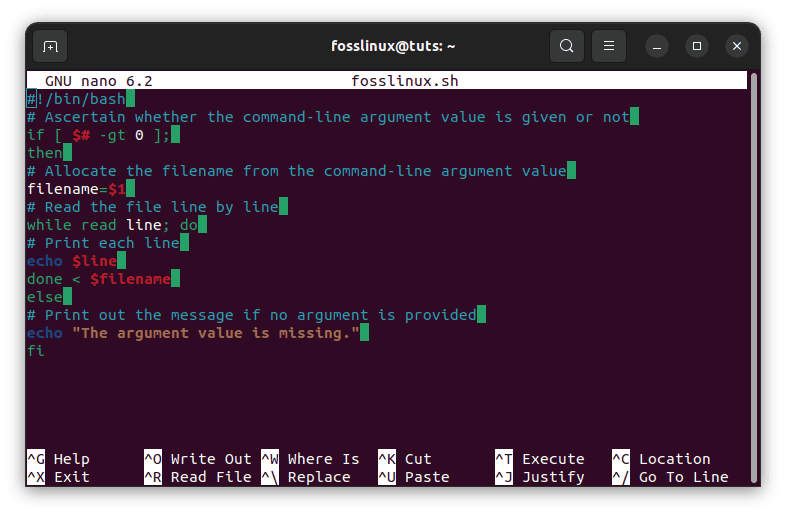
read the file line by line
Output:
When the aforementioned script is executed, the output shown below will appear:
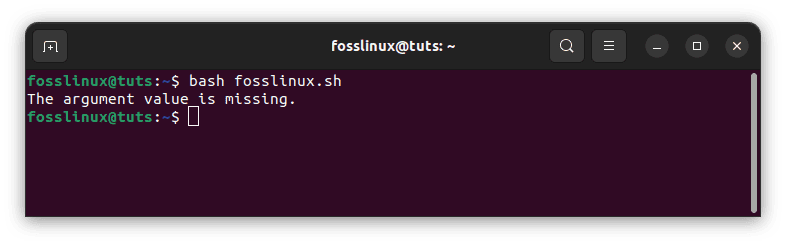
read the file line by line output
Example 9: Constructing an infinite loop
To accomplish a variety of programming goals, it is frequently necessary to create an infinite loop. To test the code of the infinite loop, create a bash file with the name fosslinux.sh and run it. In this particular illustration, the loop does not have a termination condition attached to it. An endless loop is a particular kind of loop that never ends. In this case, an exit statement is needed to get out of the infinite loop. Therefore, this loop will be iterated 10 times, and when the iteration value reaches the value 10, the exit line that allows the program to exit the infinite loop will be executed.
#!/bin/bash # Initialize the counter n=1 # Assert an infinite loop while : do printf "The current n value=$n\n" if [ $n == 3 ] then echo "Excellent" elif [ $n == 5 ] then echo "Good" elif [ $n == 7 ] then echo "Worst" elif [ $n == 10 ] then exit 0 fi # Increase the value of n by 1 ((n++)) done # Orate the created filename read filename # Read the file's content from the terminal while read line do echo $line >> $filename done
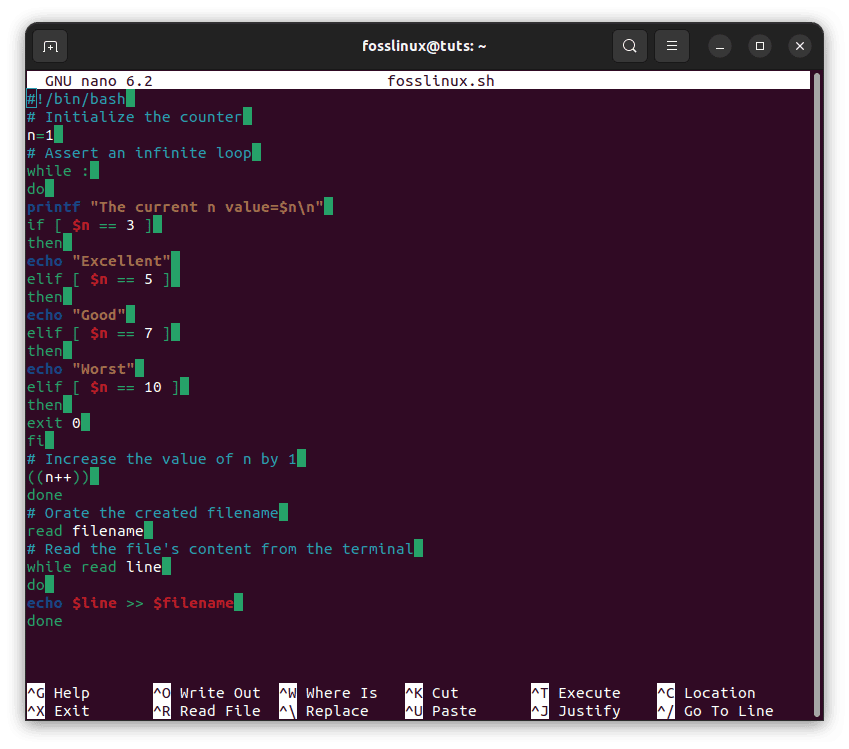
infinite loop
Output:
When the aforementioned script is executed, the output shown below will appear:
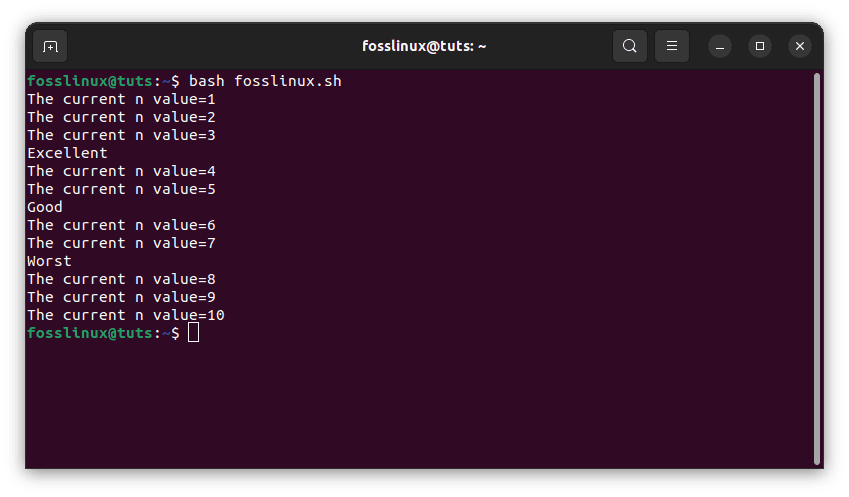
infinite loop output
Example 10: Using Multiple Conditions in the Bash While Loop
In this illustration, we will build a while loop with a compound condition consisting of several simple conditions. Logical operators are used to combine the more detailed requirements.
#!/bin/bash count=20 a=0 b=0 # multiple conditions while [[ $a -lt $count && $b -lt 4 ]]; do echo "$a" let a++ let b++ done
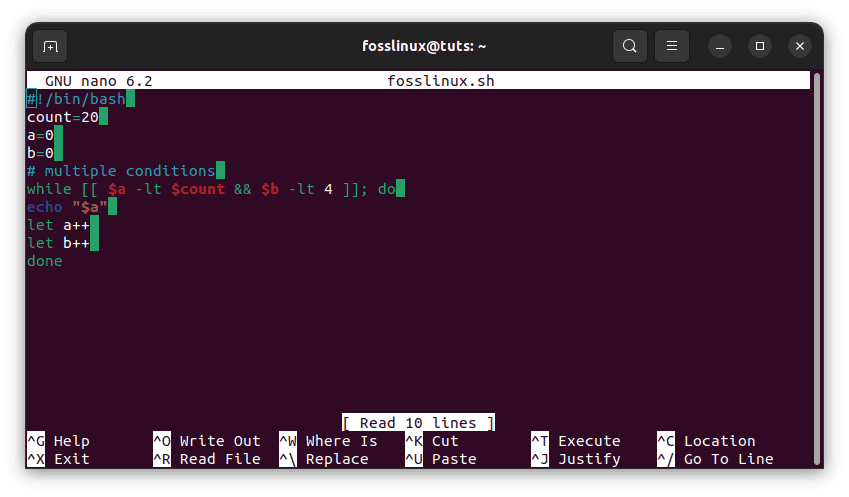
multiple conditions
Output:
When the aforementioned script is executed, the output shown below will appear:
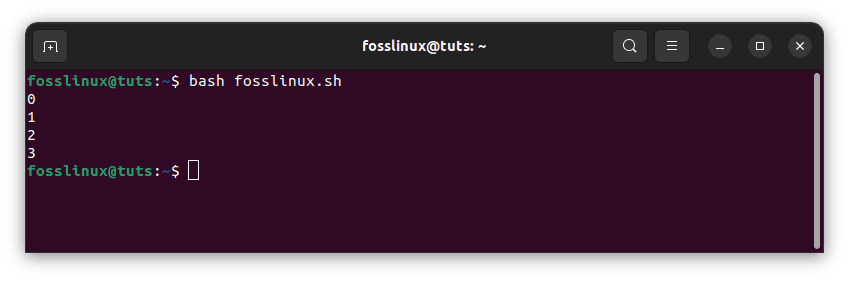
multiple conditions output
Other Examples
In addition, we can utilize the continue command to exit the while loop for the current iteration while continuing to carry out the loop’s intended functionality (as long as the condition is still true). This operates in the same manner as break, except rather than going on to the subsequent section of the script, it just repeats the previous loop.
#!/bin/bash i=0 while : do ((i++)) if [ $i -ge 6 ] && [ $i -le 19 ]; then continue fi echo Infinity Countdown: $i... sleep 0.1s done
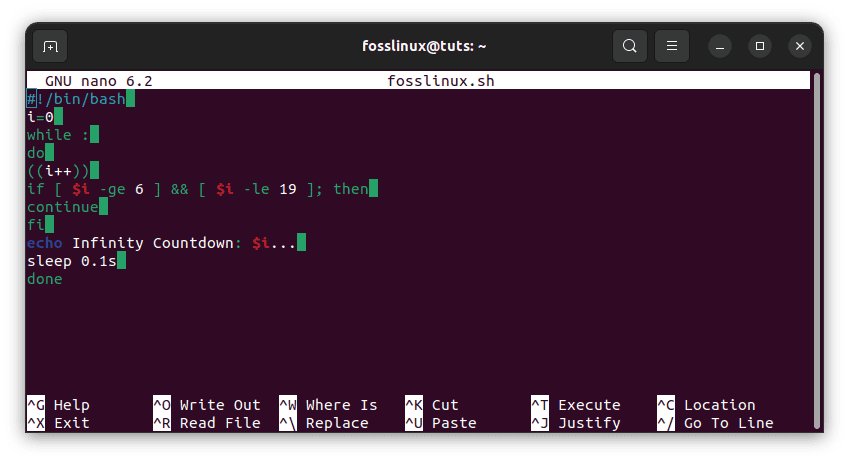
continue command to exit loop
If the value of the $i variable is between 6 and 19 at any point throughout the execution of this example, the continue statement will be executed. The output below demonstrates that our countdown to infinity timer will skip from 5 to 20 due to this action. We can exit the while loop early by using the continue command, which causes the program to revert to the beginning of the loop rather than continuing to the subsequent section of the script. When we run the script, the following is what appears on the screen of our terminal:
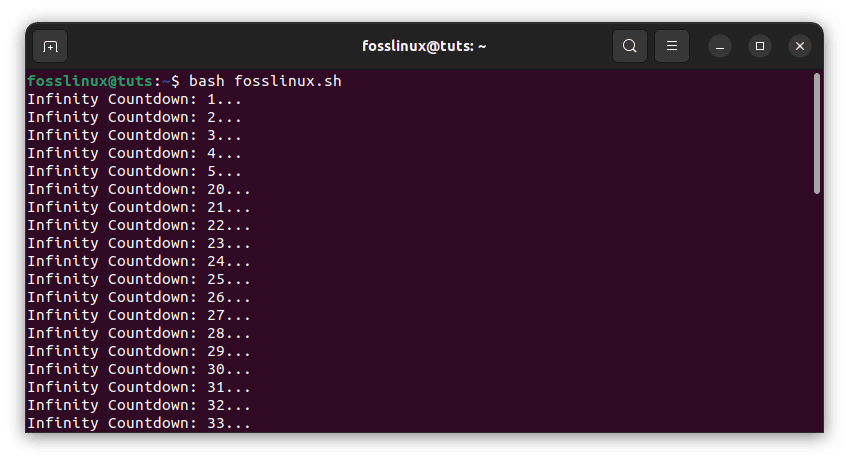
continue command to exit loop output
Within a while loop, the break command can be used to exit the loop early and abruptly halt the execution of the loop’s code. In most cases, the break statement would be implemented after a specific condition was validated, as would be the case with an if statement. Let’s go back to our “countdown to infinity” example from earlier, but this time we’ll include a break in it:
#!/bin/bash i=1 while : do if [ $i -eq 6 ]; then break fi echo Infinity Countdown: $i... ((i++)) sleep 0.1s done echo Countdown complete.
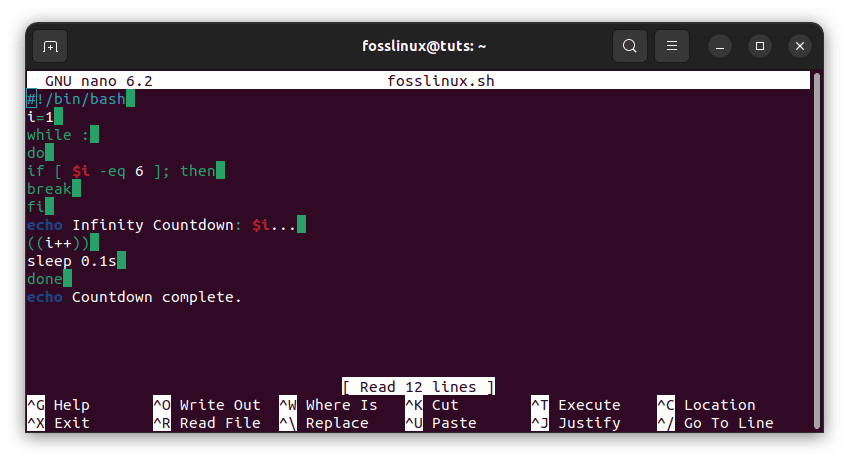
break command to exit loop
In this particular instance, our countdown will be terminated as soon as the variable is equal to 6, which should take precisely five rounds of the loop. The script will continue to whatever is written after the while loop once the break condition has been met. In this particular example, that is just an echo command. When we run the script, the following is what appears on the screen of our terminal:
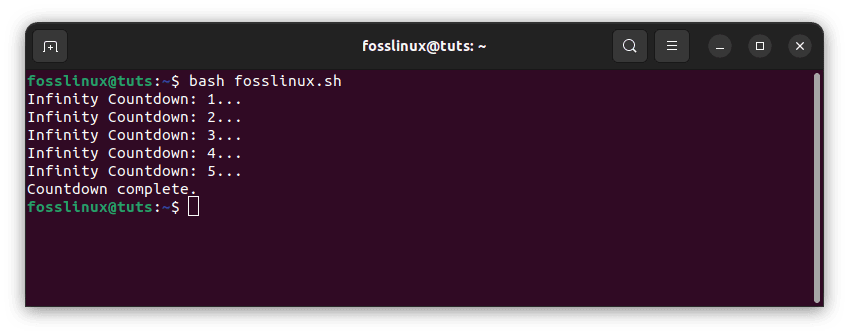
break command to exit loop output
Finally, we shall reiterate the infinite loop using the example provided below:
A loop that does not have a beginning or an endpoint is known as an infinite loop. A never-ending loop will be produced if the condition is always found to have a positive evaluation. The loop will continue to run indefinitely unless it is explicitly terminated using the CTRL+C key combination:
#!/bin/bash #An infinite while loop while : do echo "Welcome to FossLinux." done
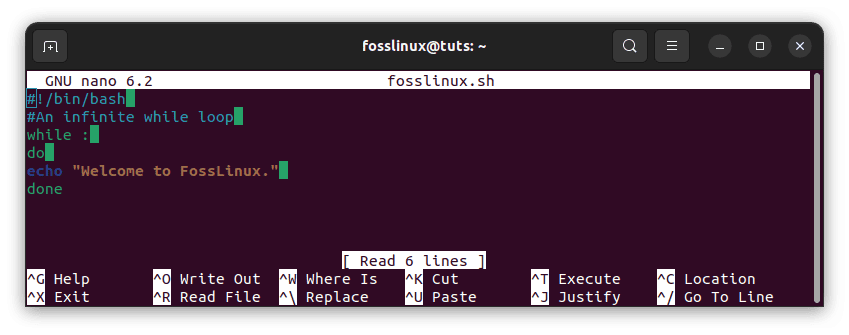
infinite loop example
The script that we just went over can alternatively be written in a single line as:
#!/bin/bash #An infinite while loop while :; do echo "Welcome to FossLinux."; done
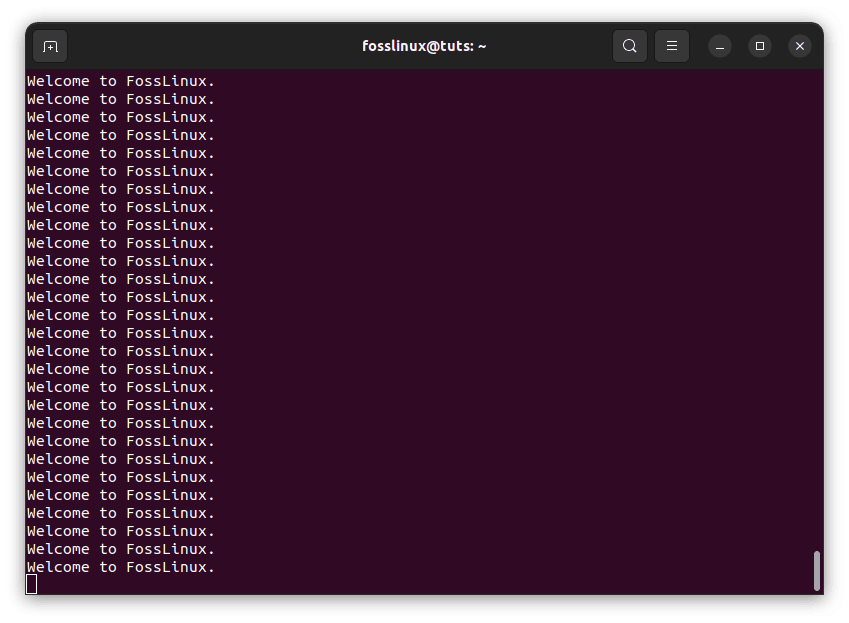
infinite loop example output
Conclusion
In this tutorial, various applications of the while loop have been broken down and illustrated with the help of several different examples. After practicing with these examples, I think a Bash user can correctly incorporate this loop into their script. A provided series of commands is carried out iteratively by the while loop so long as the evaluation of a condition yields a positive result.