Python is the fastest-growing programming language in the world. Major websites like Instagram, Pinterest, Quora, and many others are built using python’s Web Framework Django. The thing that makes python most popular is its simple syntax, which is similar to the normal English language. Its powerfulness makes it a primary choice adopted by top tech companies.
We can use python for almost every task, including web development, Automation, Artificial Intelligence, Cyber Security, Data Science, Android App Development, and various other general programming tasks. There is also huge community support for the language in Github, PyPI, and many other places, which makes it easy to solve errors, and the language is free from bugs.
Python has many libraries and frameworks written by the open-source contributors that make our task easy, and we can use them for free without reinventing the wheel. In this tutorial, we will learn the basics of python, such as reserved words, comments, variables, operators, data types, etc.
To follow this tutorial, python should be installed and configured in your system path. Many computers come pre-installed with python. You can check your python installation and version by running the following code in the terminal.
python --version
If you don’t have python installed, you can install it from python.org or see our guide here. It is recommended to have the latest version of python. If you have an older version of python, then check our tutorial on updating python to the latest version.
Python Shell
Python is an interpreted language meaning it does not have a compiler but it has an interpreter. A compiler executes all the programs at once while an interpreter executes each line one by one. This means that the python executes each line of our program one by one. Python comes with a shell that can run single lines of codes and get the output.
To run the python shell, you need to open up your terminal and then type python on it.

opening python shell
You can use this python shell to run simple python code. For example, to display the string “Hello World!” on the Terminal, we need to type the following code in the python shell.
print("Hello World!")
Output:
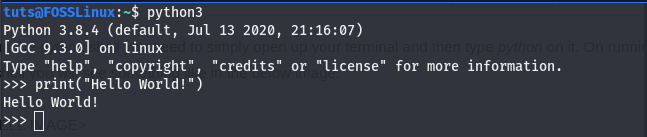
printing hello world using python shell
Now again, type the following on the shell.
1 + 5
This will display the sum of the two numbers, i.e., 6.
Output:

python shell example code
Now let us close the shell. To close the shell, we need to type exit() in the shell, as shown in the below image. For Linux users, we can also use the Ctrl+d key to exit the Shell.

closing the python shell
Using an IDE
The python shell that we discussed is a good point to start learning python and for writing small lines of codes, but it is not useful for big projects. For a real-world use case, developers use different editors to write and edit python’s code. I am using the opensource visual studio code, which is an amazing IDE for writing codes. IDE or integrated development environment is a software in which we can edit and run the code. Hence, it is great for faster and easier developments. If you want to choose between IDE for writing code, you can see our article on Top 10 IDE to be used for programming. You can use any of the IDE that you are comfortable with for writing python codes.
You can also manually write the code in a python file (having .py extensions) from a simple editor and can run it using the python interpreter by typing:
python filepathname
Where the filepathname should be the name of the file which we are using. To do this, you don’t need any installation of IDE as you are writing code on your editor.
Now, let us start learning the basics of python. I assume that you are using any of the above mentioned methods for writing and running the python codes.
Basic Python Syntax
The Python syntax is one of the most awesome things for anyone using python. The python language is a high-level language. Its syntax is very similar to the natural English language, making it easy to read and adoptable by both beginners and experts. For example, let us see the HelloWorld example in python run the below code in your python IDE or python shell.
print("Hello World!")
The above code will print the string Hello World! in the Terminal screen. On running the code, we will get the following output.

the basic syntax of python
As you see in the code, there were no semicolons at the end of the statement. This is a benefit in python as its the programmer choice whether to include semicolons or not at the end of statements.
If you come from a background in programming languages like C or C++, you may notice that if we don’t give semicolons, sky will fall on your head and syntax error will occur. But while writing multiple statements in one line, we need to use semicolons. The python code also doesn’t use curly braces for code blocks that other programming languages do; it only depends on your code’s proper indentation.
Keywords
Keywords were reserved by the python language and have some special meaning for the python interpreter. Those keywords are special words, and we cannot use them as identifiers’ names. To get the complete list of keywords in python, open up your python shell and run the following command.
help("keywords")
This will list all the keywords present in python.
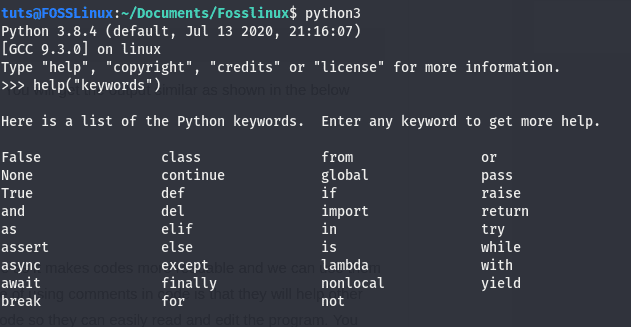
keywords in python
Comments in Python
Comments are critical while writing code as it makes codes more readable. Also, we can use them to leave remarks in our code. The main purpose of using comments in code is to help other programmers know what is going on in the code to easily read and edit the program. You can also benefit from leaving comments in the code for yourself as it can get confusing when you try to edit it in a prolonged future. Python treats the comments as white space and doesn’t execute it.
There are two types of comments that we can write in python they are single line and multi-line comments. After the # symbol is treated as single-line comments, anything after # on that line will be treated as whitespace by the python interpreter. For example, see the below code.
# this is first comment print("Hello World!") # this is second comment
On running the above code, you will see the string Hello World! printed on the screen without any error because everything else written after # are comments in the program.
We can also write comments in multiple lines. We need to write the comments under three quotes (either single or double). Look at the below code, for example.
""" This is a multi line comment. We an extend this comment to any number of lines"""
Data Types in Python
There are several types of data types. Let us discuss some of the most used data types in python.
- Integer: Like many other programming languages python also have support for integer data type they include all the negative, positive, and zero number like -1, 0, 9, etc.
- Float: The float data types include the floating-point numbers, i.e., the numbers that contain a decimal point. For example, 1.01, 1.1, 0.001, etc
- Complex Number: Python also has supports for complex numbers. For Example: 1+1j, 4+6i, etc
- String: Strings are one of the most important data types in python. They are the characters enclosed in single or double-quotes. For example, “Hello”, “FossLinux”, etc.
- Booleans: Python also has support for boolean data, i.e., True or False.
- List: Python list is an ordered collection of data that allows storing different types of data types. A list is very similar to an array in JavaScript. To create a list, we give the data inside square brackets [] separated by commas. Example of the list are:
['sam', 'david'] [1, 10, 6, 5]
- Tuple: A tuple in python is an ordered collection of different data types and similar to a list. But the difference in a tuple is that we cannot modify a tuple once it has been created, i.e., they are immutable. Tuples are created by giving the data inside brackets () separated by commas. Some example of tuples are:
('sam', 'david') (1, 10, 6, 5)
- Dictionary: The python dictionary is an unordered collection of data in a key: value pair format. We can create a dictionary by giving the key: value pairs inside curly braces {} separate by commas. For example, see the below dictionaries.
{'name':'Fosslinux', 'country':'Internet', age:5}
We can also check the data types of data/variables in python using the built-in type() function. The type() function accepts the data/variable as an argument and returns the data type of that data/variable. For example, copy and run the following code in Python IDE.
print(type(10)) print(type(10.001)) print(type("Fosslinux")) print(type(1 + 8j)) print(type([1, 10, 6, 5])) print(type((1, 10, 6, 5))) print(type({'name':'Fosslinux', 'country':'Internet'}))
Output:

checking the data type in python
Variables in Python
Variables are used to stores data in the primary memory. A variable refers to the memory address in which the data is stored. There are also some rules while selecting a variable name. The rules are given below.
- A variable name should start with a letter or an underscore character.
- A variable name cannot start with a number, or it leads to an error.
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ); no other special characters will be allowed.
- Variable names are case-sensitive, which means that var and Var are two different variables.
- The variable name should not be any of the keywords that we discussed earlier.
These rules should always be followed while declaring variables; else, the python interpreter will throw an error. The variables name can be any name that follows the above rules. Still, it is recommended to choose the name that best described the variable’s purpose and increase the code’s readability.
Operators in Python
Python programming language supports many different types of operators. In this section, we will discuss some of them.
Assignment Operators
The assignment operators are used to assign some value to a variable. We mostly used the = sign to assign data to a variable. For example, run the below code in your Python IDE.
a = 10 print(a)
You will get 10 printed on the screen on running the above code, as shown in the below code. In this code, I have used the assignment operator to assign the value 10 on the variable a and then display it using the print() function. There are many more assignment operators of which we will discuss in brief in later articles.
Arithmetic operators
These operators are used to perform arithmetic operations on two numbers: addition, multiplication, etc. There are operators like +, – , *, /, %, //, ** which can be used for arithmetic. For more details, see the following code; you can copy and run it on your IDE.
# assigning data to variables a = 10 b = 5 # adding the two numbers print( a+b ) # subttracting the two numbers print( a-b ) # Multiplying the two numbers print( a*b ) # dividing the numbers print( a/b ) # the % operator is used to get the remainder # when divided the first number from second print( a%b ) # The // operator is used to get only the integer part of the division print( a//b ) # The ** operator is used to multiply a with a, b times means a^b print( a**b )
The above code has shown you all the arithmetic operators available in python.
Output:
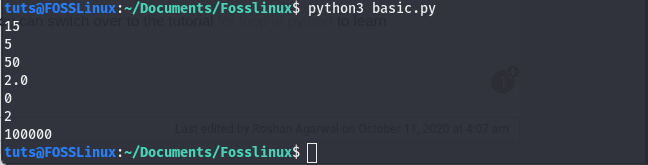
arithmetic operators in python
There are many more operators in python. In this article, I have discussed the most important operators we need to know in python. Stay tuned to FOSS Linux for tutorials on more advanced operators in upcoming articles.
Conclusion
With that we have finished the basics of python. Now it’s the best time to switch over to our next tutorial “Getting system information of Linux in Python” to learn how to use python to gather system and hardware information in Linux.