The string is one of the most popular data types in python. We can use the string data type to store any text data. In python, any character under single or double quotes is considered strings. These characters can be any of the Unicode characters that support in python. In this tutorial, we will learn almost everything about the string data type in python.
To follow this tutorial, it is recommended to have the latest python version installed in your system. If you have an older version of python installed in your system, then you can follow our guide on updating python on Linux.
Creating Strings in Python
To create a string in python, we need to put the characters’ array underquotes. Python treats both the single and double quotes as the same, so we can use any of them while creating a string. See the below examples where we create a string, store them in variables, and then print them.
# creating a string
greeting = "Hello World"
# printing the string
print(greeting)
On running the above code, you will get the output Hello World.

creating strings in python
In this code, we have created a string Hello World and stored it into a variable named greeting. Then we use the Python print function to display the string stored in the variable. You will get the following output on running the code. We can also create a multiline string using triple quotes in the below example code.
var = """Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua."""
print(var)
Here is the output.

multiline string in python
In Python, strings are arrays of bytes representing Unicode characters. But it does not have any built-in data type for single characters as in C or C++. Any string that has a length of one is considered a character.
Length of Strings
In many situations, we may need to calculate some string length. There is a built-in function that can calculate the length of a string. The function we will be using is the len() function.
To see a practical example of the len() function, run the following code in your Python IDE.
var = "This is a string"
print("The length of the string is :", len(var))
Output:

length of string using len() method
We can also use the python for loop, which I discussed in this tutorial, to calculate a string’s length.
Example:
var = "This is a string"
count = 0
for i in var:
count = count + 1
print("The length of the string is :", count)
Output:

length of string manually using for loop
String Concatenation
String concatenation is the merging or joining of two strings. We can join two strings easily by using the + operator. Let us see an example of joining two strings in python.
# creating two strings
string1 = "Hello"
string2 = "World"
# merging the two strings
greeting = string1 + string2
print(greeting)
In the above code, we have created two strings, namely “Hello” and “World,” and store them in two variables named string1 and string2. Then we used the + operator to join the two strings and store them in a variable named greeting and displayed it using the print() function.
Output:

concatenating two strings
Repetition of String
We can repeat a string multiple times in python using the * operator. For example, to print the string “Fosslinux” two times, we need to write the following code.
print("Fosslinux"*2)
Output:

repetition of strings
Formatting strings
It is effortless to do string formatting in Python. There are three ways:
1. Old formatting style
The old style of formatting strings is done using the % operator. We need to use special symbols like “%s”, “%d”, “%f”, “%.f”. with the string and then specify the tuple of data we want to format at that place. Let us see what the data accepted by the above symbols are.
- %s: It will accept strings or any other data with string representation like numbers.
- %d: It is used to give the integers data in a string.
- %f: It is used for floating-point numbers.
- %.f: It is used for Floating point numbers with fixed precision.
For example, see the below code. You can copy and run the code in your favorite python IDE.
string1 = "It is a formatted string with integer %d" %(1)
string2 = "It is a formatted string with string %s" %("Fosslinux")
string3 = "It is a formatted string with float data %f" %(1.01)
print(string1)
print(string2)
print(string3)
Output:

the old style of string formatting in python
As we can see in the output, we have formatted the strings with the integer, float, and string data. This string formatting method is the oldest way, but it is less used nowadays.
2. Using the format() method
This is a new string formatting technique introduced in Python 3. The format() functions take the data as an argument and replace them in the string where the placeholder {} are present.
Example:
string1 = "It is a formatted string with integer {}".format(1)
string2 = "It is a formatted string with string {}".format("Fosslinux")
string3 = "It is a formatted string with float data {}".format(1.01)
print(string1)
print(string2)
print(string3)
print("{} is a great website for learning {} and {}".format("FossLinux", "Linux", "Python"))
We will get the formatted string as the output on running the above code, as shown in the below image.

string formatting using format() function
3. f-strings
The latest string formatting technique is string interpolation or f-strings, introduced in python’s version 3.6. We can specify a variable name directly in an f-string, and the Python interpreter will replace the variable name with the data value corresponding to it. The f-strings starts with the letter f, and we can inject the data in their corresponding positions directly. This technique of string formatting has become quite popular in recent days. To see a demo of its working, copy the below code and run it in your python IDE.
string1 = f"It is a formatted string with integer {1}"
string2 = f"It is a formatted string with string {'fosslinux'}"
string3 = f"It is a formatted string with float data {0.01}"
print(string1)
print(string2)
print(string3)
a = "Fosslinux"
b = "Linux"
c = "Python"
print(f"{a} is a great website for learning {b} and {c}")
We used the string interpolation method for formatting strings in the above code. The strings started with the f character are f-strings. The f-string has made our work simple, and we can write the variables directly in the strings by giving variables under the {} placeholder. On running the above code, we will get the following output.

string formating using f-strings
Check for a substring
Often, we may want to check a character’s existence or a substring in a string. This can be done by using the in and not in Python keywords. For example, To check if hello is present in the string hello world, we need to run the following code.
x = "hello" in "hello world"
print(x)
On running the above code in a python IDE, we will get the boolean value True as an output, which means that the substring “hello” is present in the “hello world”.

check for a substring in a string
Let see another demonstration to know how it works in a better way.
string = "FossLinux is a great website to learn Linux and Python"
print("Fosslinux" in string)
print("FossLinux" in string)
print("Foss" in string)
print("Pyt" in string)
print("hon" in string)
print("Python" not in string)
Output:
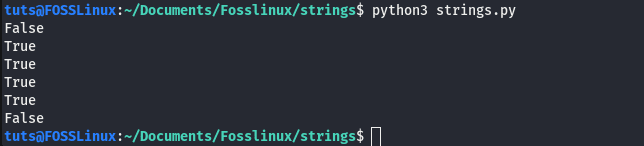
check for a substring in a string using in keyword
In the above code, we have used both the in and the not in keywords to check a substring in the parent string.
String as a sequence of Characters
The python string is a sequence of characters; they are almost similar to other python ordered sequences like list, tuple, etc. We can extract individual characters from the strings in many ways, like unpacking them using variables and indexing that I will discuss in the next topic. We can unpack the strings by assigning them to variables. To see how it works, just copy and run the following code in your favorite python IDE.
language = 'Fosslinux'
# unpacking the string into variables
a,b,c,d,e,f,g,h,i = language
print(a)
print(b)
print(c)
print(d)
print(e)
print(f)
print(g)
print(h)
print(i)
Output:
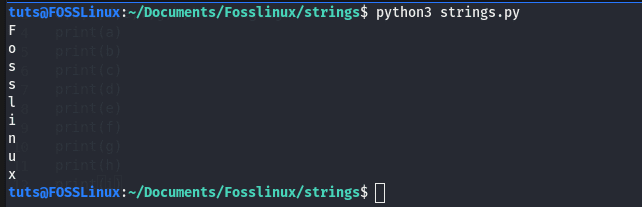
unpacking characters using variables
Indexing strings
String indexing is a fundamental and popular technique by which we can access a string’s character and perform many string operations very easily. In programming, counting starts with zero(0), so to get the first character of a string, we need to give zero in the index. To see a practical example of indexing, copy and run the following code in a Python IDE.
string = "Fosslinux"
print(string[0])
print(string[1])
print(string[2])
print(string[3])
In the above code, we first create a string named Fosslinux, and then we use the python string indexing to get the first, second, third, and fourth characters of the string. We will get the following output in the terminal on running the code.

indexing strings
Python also supports negative indexing, which is very useful where we can start counting from the right side. For example, to get the second last character of a string “Fosslinux,” we need to write the below code.
string = "Fosslinux"
print("The second last term of the string is : ", string[-2])
On running the code, we will get the second last term of the string “Fosslinux,” as shown in the below image.

negative indexing in Python strings
Getting the last term of a string
Sometimes we may want to get the last term of the string. We have two ways to do this: the first one uses the negative indexing, and the second one uses the len() function with indexing.
To get the last term of the string using negative indexing, look at the below code.
string = "Fosslinux"
print("The last term of the string is : ", string[-1])
Output:

last term using negative indexing
We can also use the len() function with indexing to get the last term. To do this, we need to calculate the string’s length, and then we need to find the character by indexing the value, which is one less than the length of the string. See the below example.
string = "Fosslinux"
length = len(string)
last_index = length-1
print("The last term of the string is : ", string[last_index])
In the above code, we first created a string and stored it in a variable named string. Then we calculate the string’s length using the len() method. As indexing in python starts with zero, we need to subtract one from the length. Then we pass it as an index to the string. Thus we get the last character of the string.
Output:

last term by calculating the length
Slicing Strings
In Python, we have a great technique, an extended form of indexing known as string slicing. This can be used to slice a string into a substring. To do the slicing, we need to give the index number of the first character and last character of the substring in the string’s index by putting a semicolon in the middle of them. For a practical demo, see the below example code.
string = "Fosslinux"
print(string[1:6])
print(string[0:4])
Output:

slicing strings
Skipping Characters On Slicing
We can also skip characters while slicing a string. During slicing a string, we have the following syntax.
string[start:stop:step]
The start and stop are the default index numbers we used in the above syntax until now. The step parameter accepts an integer, which is used to give the number of characters to leave in each step.
Reversing strings
We can reverse a string easily using the slicing method. For example, see the below code. Copy the below code in your Python IDE and run it.
string = "Fosslinux"
print("The reverse string of", string,"is",string[::-1])
This code will reverse the string “Fosslinux.” On running the code, we will get the following output.

reversing string
Escape Character in Strings
Escape characters in programming is a great way to add non-printable characters in strings. For example, to add the new line character in strings, we use the escape character “\n”. See the below code for a demo.
print("\n\n\n Hello\n\n World")
On running the code, we will get the following output.
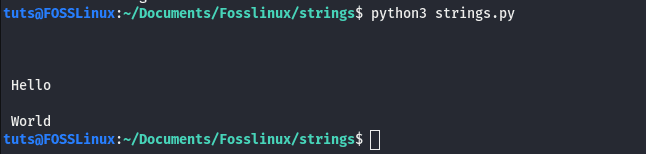
escape characters in strings
As we can see in the code that newlines are automatically added in place of “\n”. That is where the escape sequence comes into play. There are many sequence characters present in python. I will list them all here; you can try all of them to see how each works.
- \’: It is used to give a single quote in a string. As in some places, we can’t give single quotes directly.
- \\: This character is used to represent backslash, as, in many places, we can’t use \ directly.
- \n: This character represents the new line character, which will add a new line.
- \r: represents a carriage return.
- \t: represents a tab.
- \b: represents a backspace character.
- \f: This escape sequence is used to represent a formfeed.
- \ooo: This character is used to represent octal value.
- \xhh: This character is used to represent the Hexadecimal value.
- \a: This character is used to give am alert.
- \s: This character is used to give a space.
- \v: represents a vertical tab.
String Methods
We have learned many things about python strings, but this part is way more helpful than any other part of this article. Python comes with a large number of built-in functions for working with strings. By using them, we can easily perform many operations on strings.
Transforming String Cases
We have some built-in functions which can be used to transform the string cases. Let us discuss them all.
string.capitalize()
This method is used to capitalize on the target string. When we use the method as string.capitalize, it will return the string by capitalizing it, i.e., transforming the first character into uppercase and all the other characters into lowercase. To see a practical demo of its working copy and run the following code in your Python IDE.
string = "fosslinux"We used the capitalize() method of the String object, capitalizing it. On running the code, we will get the following output.
print(string.capitalize())

capitalizes a string
.upper()
This method is used to transform a string into uppercase, i.e., capitalizes all the characters present in the string.
Example:
string = "Fosslinux"
print(string.upper())
Output:

transforming a string to an uppercase
string.lower()
This method is used to transform a string into lowercase, i.e., changes all the characters present in the string to lowercase.
Example:
string = "FOSSLinux"
print(string.lower())
Output:

transforming a string to an uppercase
string.swapcase()
This is a great method for swapping the case of characters of a string. It converts the lowercase characters to uppercase and vice versa of the string. To see its working, just copy and run the following code.
string = "FOSSlinux"
print(string.swapcase())
Output:

swapping case of python string
string.title()
Again, this is an excellent method in string manipulating as it transforms the first character of every word present in the string to uppercase.
Example:
string = "Fosslinux is a great"
print(string.title())
Output:

transforming string to title
You may have noticed the difference between the capitalize() and the title() method. The capitalize() method only capitalizes the first character of the first word of the string, while the title() method capitalizes the first character of every word present in the string.
Character Classification
We also have methods to check a string’s case, whether uppercase, lowercase, etc. Let us discuss them in brief with examples.
string.isalnum()
This method is used to check whether a string contains only alphanumeric numbers or not, i.e., all its characters must be numbers or alphabets but no other characters, including whitespace.
Example:
string1 = "Fosslinux123"
string2 = "Fosslinux is a great"
string3 = "Fosslinux @ # 123"
print(string1.isalnum()) # contain only alphabet and numbers
print(string2.isalnum()) # contain whitespace
print(string3.isalnum()) # contain special characters
Output:

checking for alphanumeric numbers in a string
string.isalpha()
This string method is similar to the above method, but it checks only for alphabets, not the numbers i the string, which means the string must only contain alphabets. For example, run the following code.
string1 = "Fosslinux123"
string2 = "Fosslinux"
print(string1.isalpha()) # contain alphabet and numbers
print(string2.isalpha()) # contain only alphabet
We will get False for the first one because it contains numbers, and we get True for the next one as it contains only alphabets.
Output:

checking for alphabets in strings
string.isdigit()
This method is similar to the above one, but instead of alphabets, it checks if the string consists of only digits. It returns True if every character present in a string are digits; else returns False.
string.isidentifier()
This is also a great string method of python. By using this method, we can check if a string is a valid python identifier or not. I have discussed the rules for choosing the valid python identifier in the basics of the python tutorial.
Example:
string1 = "Fosslinux123"
string2 = "123Fosslinux"
string3 = "_Fosslinux"
string4 = "Fosslinux@1234"
print(string1.isidentifier()) # True
print(string2.isidentifier()) # False (started with numbers)
print(string3.isidentifier()) # True
print(string4.isidentifier()) # False (contain special characters @)
Output:

checking for identifier in string
string.islower()
This string method checks if all the string characters are lowercase. If yes, it returns True else returns False.
string.isupper()
This string method checks if all the characters present in a string are uppercase. If yes, then it returns True else returns False.
string.istitle()
The istitle() method of the string will return True if the first alphabet of all words present in a string is uppercase, and all other characters are lowercase.
string.isprintable()
It returns True if all the characters present in the string are printable, i.e., non-escape characters; else, it returns False. To see how it works, run the following code.
string1 = "Fosslinux"
string2 = "\nFosslinux"
print(string1.isprintable()) # True
print(string2.isprintable()) # False (It contains the new line character)
Output:

checking for printable characters
string.isspace()
The string.isspace() method will return True if all the string characters are white space characters; else, it will return False.
Other Important Functions
string.count()
The count() method of the String object is used to get the number of times a specified value occurs.
Example:
string = "Fosslinux"
print(string.count("s"))
In the above code, we used the count() method to get the number of times the character “s” appears in the string “Fosslinux.”
Output:

counting specified characters in string
string.startswith()
This string method checks if the string starts with the substring given in the method’s argument. To see a practical demo of its working, copy and run the below code in a Python IDE.
string = "Fosslinux"
print(string.startswith("F"))
print(string.startswith("Fo"))
print(string.startswith("Foss"))
print(string.startswith("Fosss"))
On running the above code, we will get True for the first three while the last one returns False, as shown in the below output image.

the string starts with
string.endswith()
This is similar to the above method, but the difference is that while the previous one check for the start of a string, it will check at the end of the string.
string.find()
The find() method of the String object is an important method to find a character or substring in a string. It accepts the substring as an argument and returns the substring index if present in the string; else returns -1.
Example:
string = "Fosslinux"
print(string.find("lin"))
On running the above code, we will get the output as 4, which is the starting index of the substring “lin” in “Fosslinux.”

string find function
string.replace()
The syntax of this method is replace(old, new). It takes two arguments; one is the old substring, and the new is the substring. It replaces all the old substring with the new substring in the entire string.
Example:
string = "Fosslinux"
print(string.replace("Foss",""))

string replace function
string.split()
This method takes the separator as an argument, splits the string according to the separator, and returns a python list.
Example:
string = "Fosslinux is a great place to start learning linux and python"
print(string.split(" "))

python string split function
string.strip()
This method is used to remove all the leading and trailing whitespaces from the string.
Conclusion
That’s all about Strings and its usage in Python. Going through the tutorial will give you an idea of how useful it is working with strings in python. You may also want to see the tutorial on using for loop in python, an ultimate loop for iteration in python. Finally, before we leave, you might want to look at the method to reverse a string in Python, which comes in handy while handling strings.