In this tutorial, we will learn about the processing that can be done with numbers in python. To work with this tutorial, it is recommended to install the latest version of python. You can refer to our tutorial for installing the latest version of python on Linux. If you use other operating systems, switch to python official website and download a binary from there.
Python Tutorial: Working with Numbers
It is also recommended to choose a python IDE for writing python code. Using the VS code, you can use it or choose an IDE from our top IDE listing.
Introduction
It is straightforward to work with numbers as python itself is a simple and powerful language. It supports three numeric types, namely:
- int
- float
- complex number
Although int and float are common numeric data types present in many programming languages, the support for complex numbers by default is a python’s unique capability. Let us see details on each of these numbers.
Integers and Floating-Point numbers
In programming, integers are a number with no decimal point, For example. 1. 10. -1, 0, etc. While the numbers with decimal points like 1.0, 6.1, etc. are called floating-point numbers or float.
Creating Integers and Floating-Point Numbers
To create an integer, we need to assign the integer value in a variable. For illustration, see the code below:
var1 = 25
In this code, we assign the integer value 25 in a variable named var1. But remember not to use single or double quotes while creating numbers as it represents the number as string data type instead of integers. For example, look at the below code.
var1 = "25" # or var1 = '25'
In writing with quotes, the data is represented as a string but not a number due to which we can’t process it.
To create a number with the float data type, we need to assign the value into a variable, as I did in the following line of code.
var1 = 0.001
Like integers, we must not use quotes while creating a variable here, as I discussed above.
We can also check the data type of a variable or data using python’s built-in type() function. To see a quick demo of this function, copy and run the following code in a Python IDE.
var1 = 1 # creating an integer var2 = 1.10 # creating a float var3 = "1.10" # creating a string print(type(var1)) print(type(var2)) print(type(var3))
In the above code, we used the type() function to get the data type of some variables and then display them using the print function.
Output:

getting the data type of variable
We can also create large numbers in python, but we need to remember that we can’t use comma(,) while creating numbers as I did in the following code.
# creating 1,000,000 var1 = 1,000,000 # wrong
On running the above code using a python interpreter, we will get an error because we use a comma in the integers data. To separate integer values, we need to use underscore(_) instead of a comma. Here is the correct usage.
# creating 1,000,000 var1 = 1_000_000 # right
On running the above code, it will run without any error. We can also print to check the data as I do in the below example code.
# creating 1,000,000 var1 = 1_000_000 # right print(var1)
Output:

using comma notation while writing numbers
Arithmetic operations on integers and floating-points
Let us see some arithmetic operations like addition, subtraction that we can perform on numbers. To run the example codes, open up your python shell by typing python or python3 in your terminal, as I did in the following image.
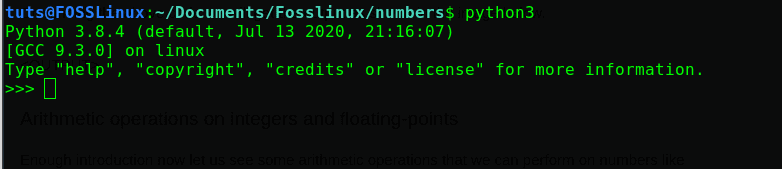
python shell
Addition
In python, addition is done by using the + operator. Open up the python shell and run the following.
>>> 1+3
We will get the sum of the two numbers printed in the Terminal, as shown in the below image.

sum of two integers
Now run the following code in the shell.
>>> 1.0 + 2
On running the above code, I added a floating-point number and an integer. You may notice that it displays a floating-point number. Thus adding two integer results in integer but adding two floats or one float and one integer would lead to floating-point.
Output:

sum of an integer and float
Subtraction
In python, subtraction is done by using the – operator. See the below code for illustration.
>>> 3-1 2 >>> 1-5 -4 >>> 3.0-4.0 -1.0 >>> 3-1.0 2.0
We can see that we get a positive integer on subtracting a large integer with a small integer. In contrast, on subtracting a large integer from a small integer, we will get a negative integer in normal arithmetic. We can also see that like addition in subtraction, if we use one number integer and other number floating-point, then the output will be a floating-type number.
Multiplication
To perform multiplication in Python, we need to use the * operator.
>>> 8*2 16 >>> 8.0*2 16.0 >>> 8.0*2.0 16.0
If we multiply an integer with an integer, we get an integer, and if we multiply a float number with an integer or float with float, we will get the output as a floating-point number.
Division
In python, Division can be done by using the / operator.
>>> 3/1 3.0 >>> 4/2 2.0 >>> 3/2 1.5
We may notice that unlike addition, subtraction, or multiplication, when we divide any two integers or floating-point numbers, it always displays a floating-point number.
On division, we may also take care that the number by which we dive must not be zero, or python will show a ZeroDivisionError. See the below code for illustration.
>>> 1/0 Traceback (most recent call last): File "<stdin>", line 1, in <module> ZeroDivisionError: division by zero
Integral Division
While dividing using the division(/) operator, we will get the exact result in the decimal point. But sometimes, we require only the integer part of the division. This can be achieved by using the integral division(//) operator. See the Python Shellcode below.
>>> 2//1 2 >>> 4//3 1 >>> 5//2 2
You may notice that we get the quotient part of the division by using this operator. We can also get the remainder of the division by using the modulus operator, which I discuss below.
Modulus
To get the remainder of two numbers, we use the modulus(%) operator.
>>> 5%2 1 >>> 4%2 0 >>> 3%2 1 >>> 5%3 2
We can see from the above code that the remainder has been clearly displayed without any error.
Exponent
We can give a number to the power of a number using the ** operator.
>>> 3**2 9 >>> 2**4 16 >>> 3**3 27
We can see that it had easily raised an integer to the power of a number.
Complex Numbers
Complex numbers are numbers containing the imaginary part. Python has native support for the complex number. We can easily create them and use them in python.
Example:
# creating the two complex numbers var1 = 2+2j var2 = 3+4j # adding the two complex numbers sum = var1 + var2 print("The sum of the two complex numbers is : ", sum)
We have created two complex numbers, which are of the form a+bj. Then we added the two complex numbers using the + operator and displayed the sum using the print() function.
Output:

sum of two complex numbers
Type conversion
Type conversion is the method of converting a number from one data type to another. We can easily convert a number from one type to another using function like float(), int(), complex().
x = 1 # creating a integer y = 2.0 # creating a floating-point number z = 2+3j # creating a complex number a = float(x) # converting integer to float b = int(x) # converting float to integer c = complex(x) # converting integer to complex d = complex(y) # converting float to complex print(a, type(a)) print(b, type(b)) print(c, type(c)) print(d, type(d))
Output:
We can see how the numbers have been changed to the desired type using simple python functions.

type conversion
Random Numbers
Random numbers may be used for creating games, in cryptography, etc. Python does not have any built-in function for generating random numbers, but it has a built-in module named random, which can be used to work with random numbers. Let us see a simple demo of generating random numbers using this module.
import random print(random.randrange(1, 1000))
Output:
We will get a new number generated between 1 and 1000.
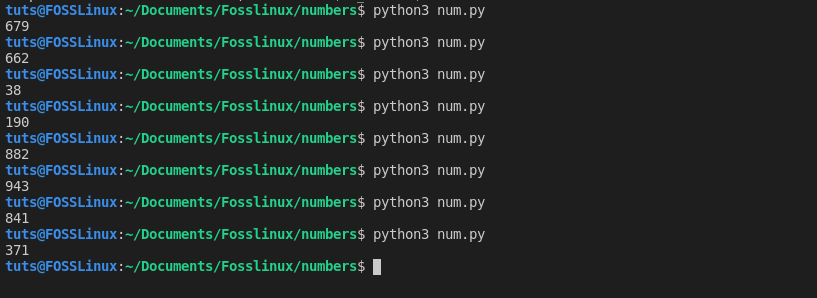
random numbers
Built-in Mathematical Functions
Python also has a wide range of built-in functions to work with numbers. Let us discuss some of the important functions.
round()
The round() function is used to round a floating-point number to its nearest integral number. While it converts the floating-point number to the nearest integer, the data type does not change. The integral number is also of the float data type.
Example:
# creating the numbers a = 0.01 b = 1.45 c = 2.25 d = 3.7 e = 4.5 # rounding the numbers print(round(a)) print(round(b)) print(round(c)) print(round(d)) print(round(e))
In the output, we can see that all the floating-point numbers have been rounded to the nearest integral value on running the code.

rounding numbers
abs()
The abs() function is used to generate the absolute value of a number. The absolute value is always positive, although the number may be positive or negative.
Example:
# creating the numbers a = 1.1 b = -1.5 c = 2 d = -3 e = 0 # displaying the absolute value print(abs(a)) print(abs(b)) print(abs(c)) print(abs(d)) print(abs(e))
Output:

absolute value of numbers
pow()
The pow() function is used to raise a number to a power. We have learned to raise the power of a number using the ** operator. This function can also be used to achieve that result.
The pow() function required two arguments, The first argument is the base number of which we want to raise the power, and the second argument is the power.
Example:
base = 8 power = 2 print(pow(base, power))
Output:
We raise the power of the base 8 to 2.

raising an integer to a power
The math Library
Python comes with a full-fledged library that can perform almost every mathematical operation; this is the math library. This python module is present in the python standard library, so we don’t need to do anything. The math module comes with some mathematical constants like PI, e, etc., and also has some useful mathematical methods like log(), exp(), sqrt(), trigonometric functions, etc.
While I plan to cover the math module in a future article, for now, you can switch to the math library’s official documentation for more details on how to use it.
Conclusion
In this tutorial, we have learned the basics of working with numbers in python. These basics will help you to perform many types of mathematical operations while writing code in python. You may also want to see our step by step guide on working with strings in python, which will increase your knowledge of the most used data type of python.