Java is a high-level programming language that is used to build enterprise-level applications. It is an object-oriented language developed by James Gosling at Sun Microsystems in the mid-1990s. Java is used extensively in various fields, including web, mobile app, and game development. In this article, we will be discussing how to run Java from the command line in Linux.
Running Java applications from the command line is a valuable skill to have. It allows you to quickly test and run Java programs without needing an Integrated Development Environment (IDE). By learning how to run Java from the command line in Linux, you will be able to improve your productivity as a developer and have a better understanding of how Java works.
This article aims to provide an in-depth guide on running Java programs from the command line, covering the installation process, compiling and executing Java code, configuring environment variables, and troubleshooting common issues. We will explore different methods to run Java from the command line in Linux. Throughout the article, we shall also discuss the installation process for Java on various Linux distributions, so you can start running Java on your Linux machine immediately.
Installing Java in Linux
The installation process for Java on Linux varies depending on your distribution. In this section, we will discuss the installation process for Java on some of the most popular Linux distributions.
First, let’s explore the steps for installing Java on a Linux system. While various Linux distributions have different package managers, we’ll cover the most common ones: apt for Debian-based systems (e.g., Ubuntu), dnf for Fedora, yum for CentOS, and pacman for Arch Linux. Next, open your terminal and execute the following commands for your specific Linux distribution:
Debian, Ubuntu, and other derivatives
To install Java on Ubuntu and derivatives, you can use the default-jdk package. Run the following command:
sudo apt-get update sudo apt-get install default-jdk
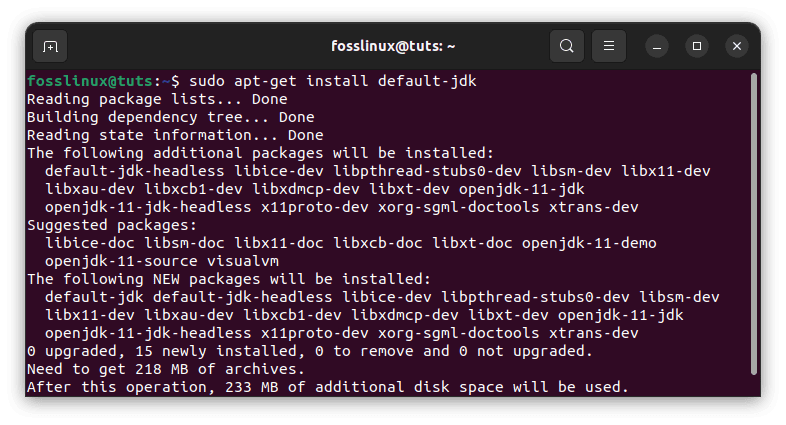
Install default jdk package
Fedora
To install Java on Fedora, you can use the OpenJDK package.
sudo dnf update sudo dnf install java-11-openjdk-devel
CentOS
To install Java on CentOS, you can use the OpenJDK package.
sudo yum update sudo yum install java-11-openjdk-devel
Arch Linux
To install Java on Arch Linux, you can use the OpenJDK package.
sudo pacman -Syu sudo pacman -S jdk-openjdk
Compiling Java programs in Linux
Once Java is installed, we can compile Java programs from the command line. Let’s consider a simple “FossLinux.java” program as an example. Open your favorite text editor and create a new file with the following content:
public class FossLinux { public static void main(String[] args) { System.out.println("Hello, Foss Enthusiasts!"); } }
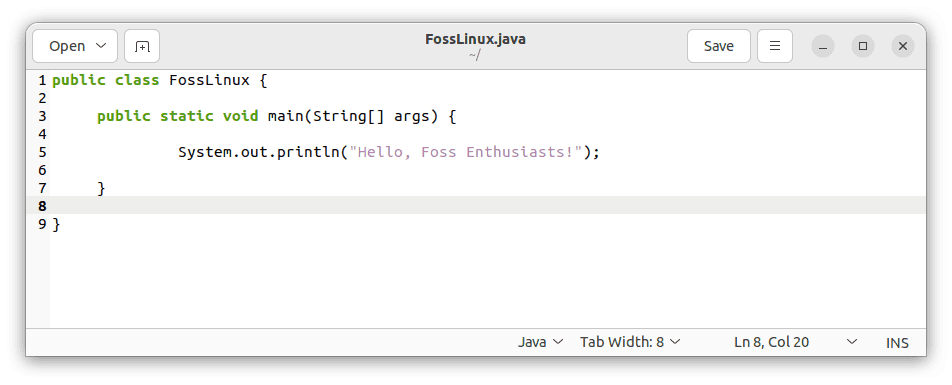
Create a FossLinux.java file
Save the file as “FossLinux.java” and navigate to its directory in the terminal. Execute the following command to compile the program:
javac FossLinux.java
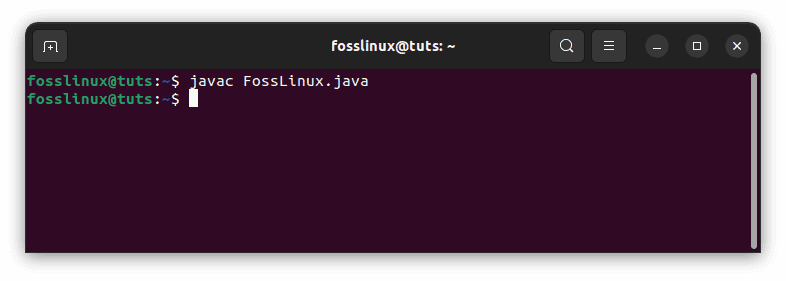
Compile FossLinux.java program
A new file named “FossLinux.class” will be generated if the compilation is successful.
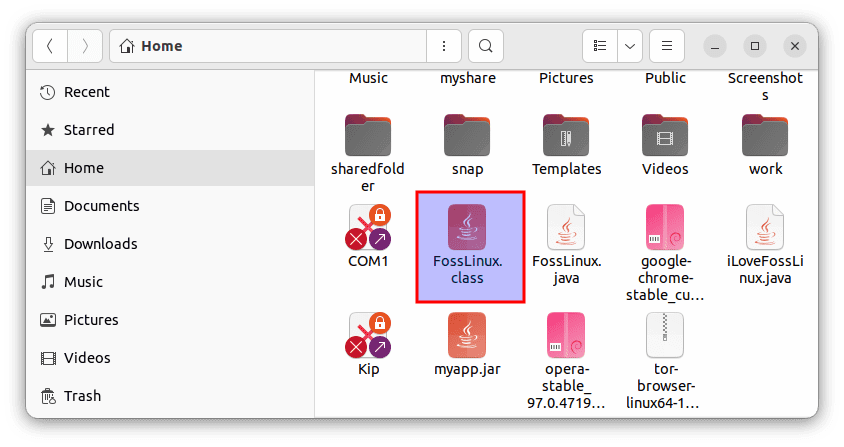
FossLinux.class file
Executing Java programming Linux
Now that our Java program is compiled let’s run it from the command line. In the same directory, execute the following command:
java FossLinux
You should see the output: “Hello, Foss Enthusiasts!”.
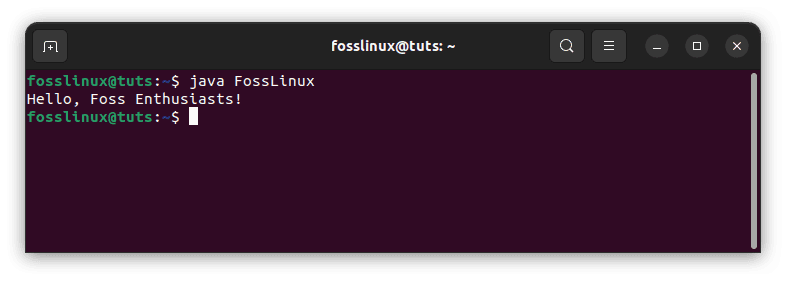
Execute FossLinux.java program
Setting environment variables
We need to set up environment variables to ensure Java programs can be executed from any directory. Let’s configure JAVA_HOME and PATH variables permanently. Open a terminal and run the following commands:
echo 'export JAVA_HOME="/usr/lib/jvm/default-java"' >> ~/.bashrc echo 'export PATH="$PATH:$JAVA_HOME/bin"' >> ~/.bashrc source ~/.bashrc
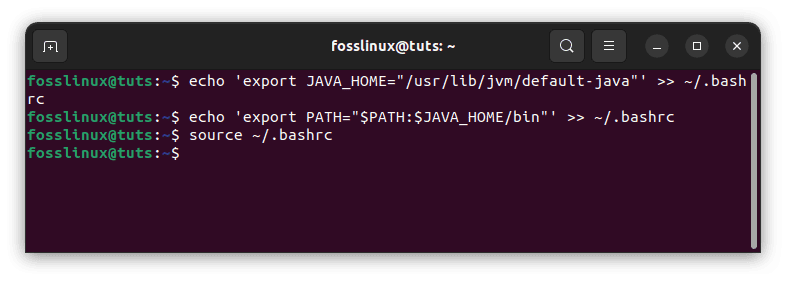
Set variables
That’s it. Java programs can now be executed from any directory.
Methods to run Java from the command line in Linux
Below are some ways that can be employed to run Java from a command line in Linux:
Method 1: Using the java command
The most basic way to run Java from the command line in Linux is by using the java command. The java command is used to launch the Java Virtual Machine (JVM) and execute Java programs.
You must install your machine’s Java Development Kit (JDK) to run a Java program using the java command. You can check whether Java is installed on your Linux machine by running the following command:
java -version
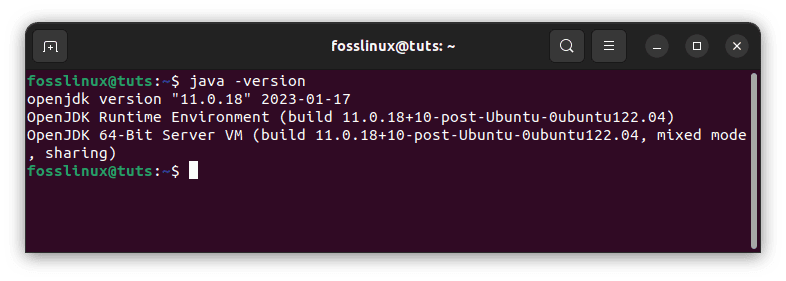
Check java version
Once Java is installed, you can run a Java program by navigating to the directory that contains the Java program and running the following command. If not, use our previous section guide to install Java on your system.
java MyProgram.java
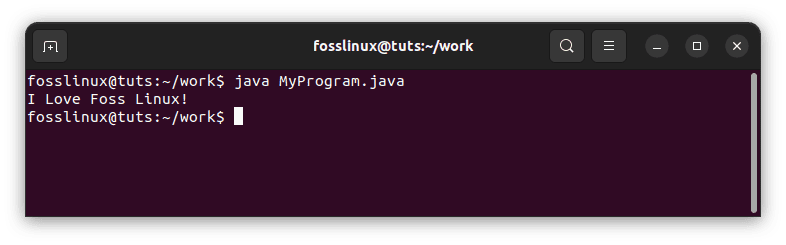
Run MyProgram.java program
Replace MyProgram with the name of your Java program.
Method 2: Compiling and running a Java program
Another way to run Java from the command line in Linux is by compiling and running a Java program. You must install the JDK on your machine to compile a Java project. Once you have the JDK installed, you can compile a Java program by running the following command:
javac MyProgram.java
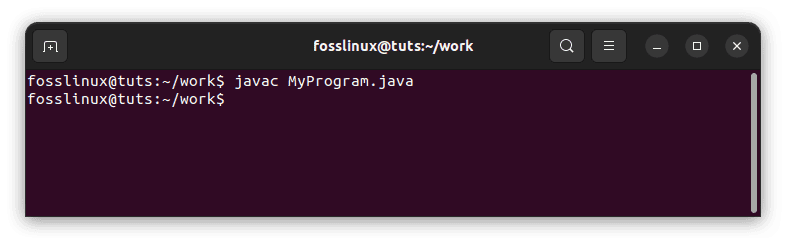
Compile a Java program
Replace MyProgram with the name of your Java program. The terminal typically does not display any output if the compilation is successful.
This command will compile your Java program and generate a class file. You can then run the compiled program by running the following command:
java MyProgram
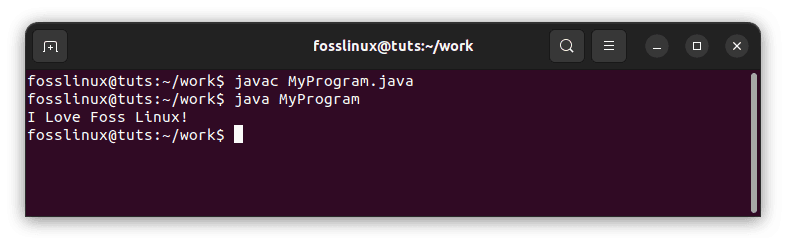
Run the compiled program
Method 3: Using an IDE
An Integrated Development Environment (IDE) is a software application that provides a comprehensive environment for developing software. IDEs are designed to simplify the development process by offering features like code completion, debugging, and refactoring.
Most IDEs have built-in support for running Java programs from within the IDE. To run a Java program using an IDE, you must create a new project, create a new Java class, and write your code. Once you have written your code, you can run the program by clicking on the run button within the IDE.
Examples
Here are a few examples that you can try running on the terminal once you have installed Java:
1. Running a simple Java program
Create a file named Hello.java with the following code:
public class Hello { public static void main(String[] args) { System.out.println("Hello, Foss Enthusiasts!"); } }
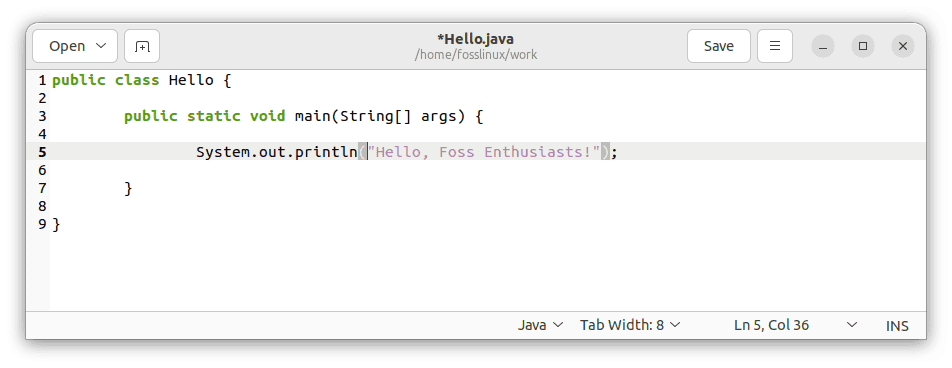
Create a Hello.java program
Compile the program using the following command:
javac Hello.java
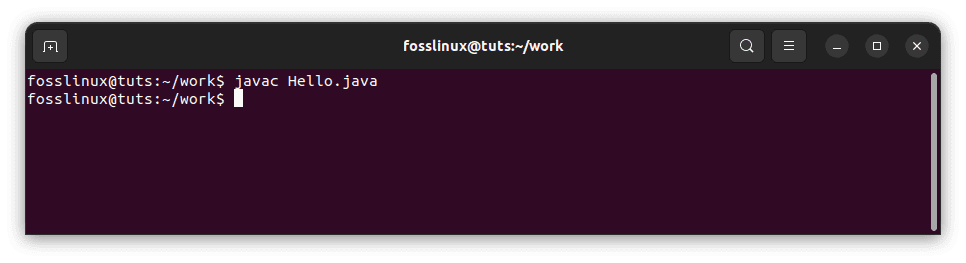
Compile Hello.java program
Run the program using the following command:
java Hello
You should see the message “Hello, Foss Enthusiasts!” printed on the terminal, as shown below.
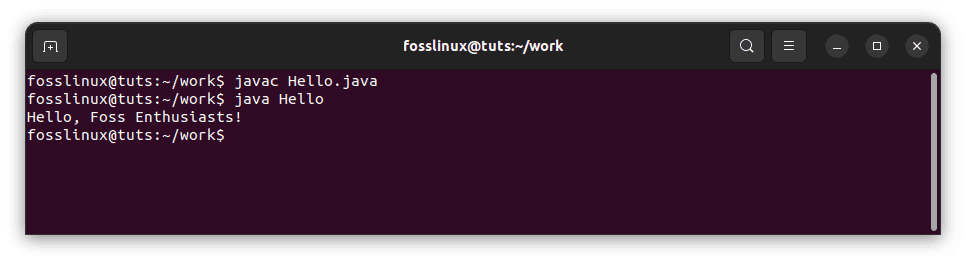
Run Hello.java program
2. Using command-line arguments
Create a file named Greet.java with the following code:
public class Greet { public static void main(String[] args) { System.out.println("Hello, " + args[0] + "!"); } }
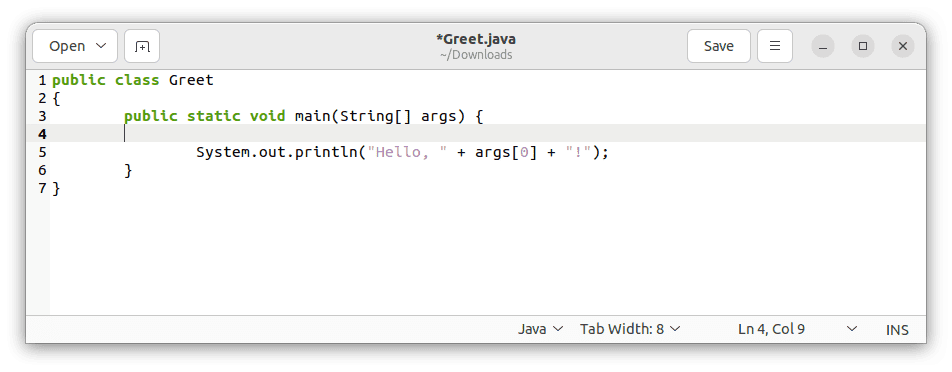
Create Greet.java program
Compile the program using the following command:
javac Greet.java
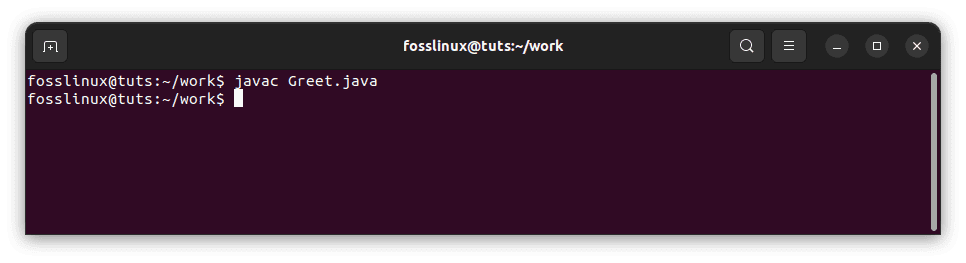
Compile Greet.java program
Run the program with a command-line argument using the following command:
java Greet FossLinux
You should see the message “Hello, FossLinux!” printed on the terminal as shown below:
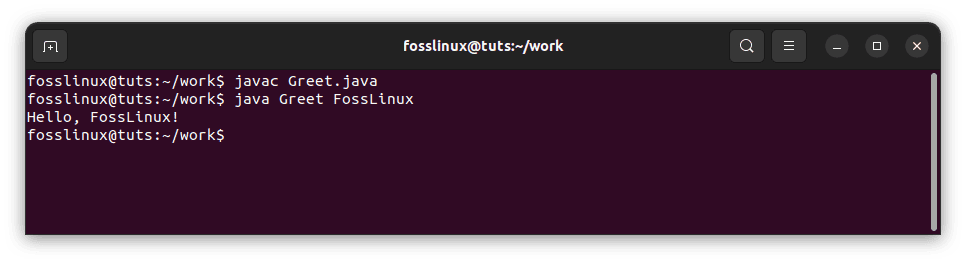
Run Greet.java program
3. Using external libraries
Create a file named LibraryDemo.java with the following code:
import org.apache.commons.math3.complex.Quaternion; class LibraryDemo{ public static void main(String[] args){ Quaternion q1=new Quaternion(1,2,3,4); Quaternion q2=new Quaternion(4,3,2,1); System.out.println(q1.multiply(q2)); System.out.println(q2.multiply(q1)); } }
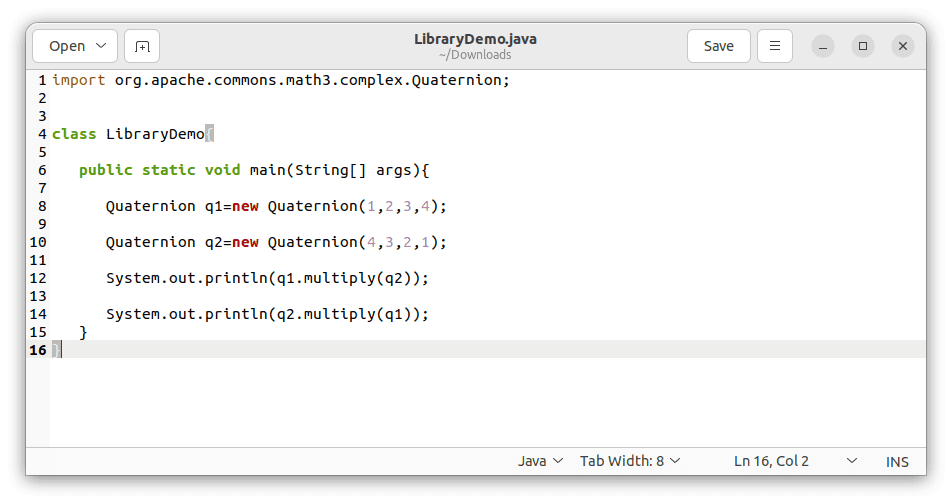
Create LibraryDemo.java program
Compile the program using the following command:
javac -cp ./commons-math3-3.6.1.jar LibraryDemo.java
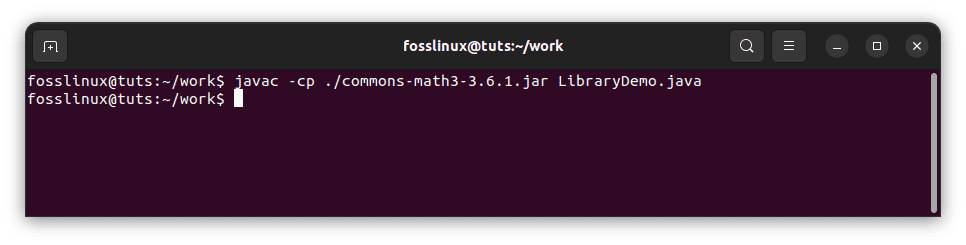
Compile LibraryDemo.java program
Note that the -cp option specifies the classpath, which includes the current directory (.) and the commons-math3-3.6.1.jar file.
Run the program using the following command:
java -cp .:./commons-math3-3.6.1.jar LibraryDemo
You should see the JSON representation printed on the terminal as shown below:
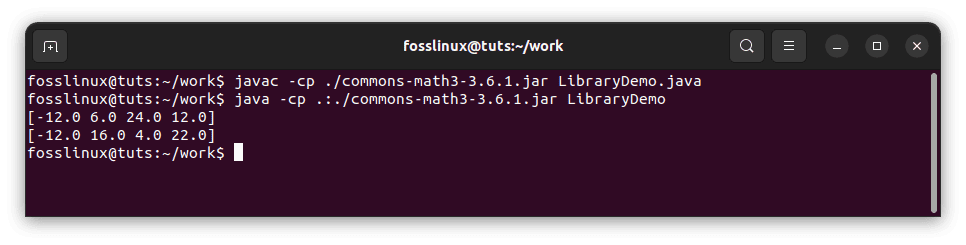
Run LibraryDemo.java program
These examples demonstrate some basic features of Java and how to compile and run Java programs from the command line in Linux. Feel free to experiment with these examples and modify them to see how they work!
Troubleshooting common issues
Below are helpful tips you can use to troubleshoot common Java issues:
1. “java: command not found”
This error occurs when the Java executable is not found in the system’s PATH. To resolve this issue, follow these steps:
Verify Java Installation: Check if Java is installed on your system by running the following command:
java -version
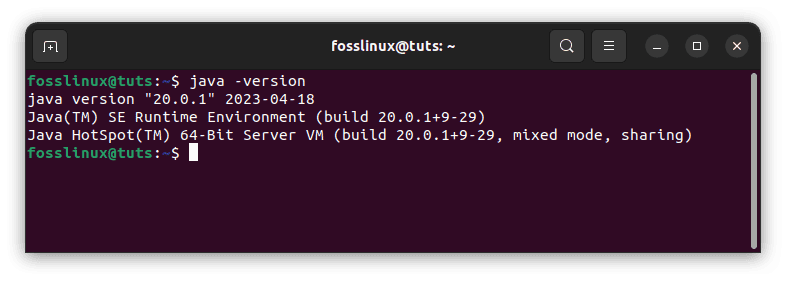
Check Java version
If Java is not installed or the command is not recognized, revisit the installation steps mentioned earlier in this article.
Verify Environment Variables: Ensure the JAVA_HOME environment variable is correctly set and appended to the PATH. Open a terminal and execute the following command:
echo $JAVA_HOME
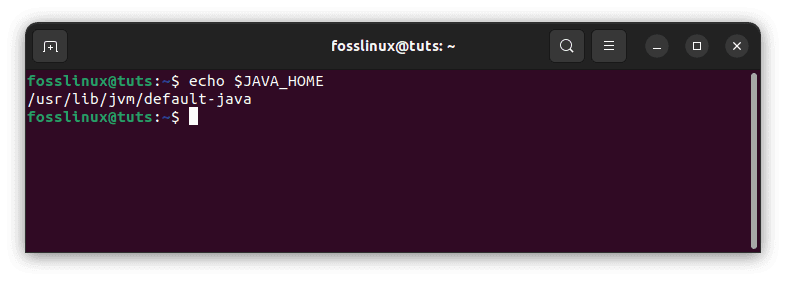
Verify environment variables
If the output is empty or incorrect, you must set the JAVA_HOME variable. Open the .bashrc or .bash_profile file using a text editor:
nano ~/.bashrc
Add the following line at the end of the file:
export JAVA_HOME="/usr/lib/jvm/default-java"
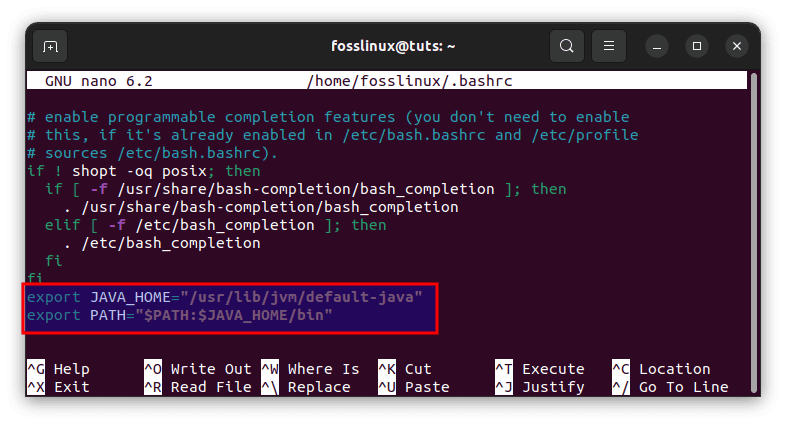
Append the following line
Save the file and exit the editor. Then, execute the following command to update the changes:
source ~/.bashrc
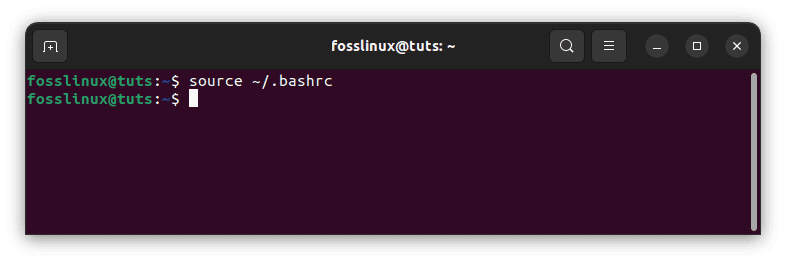
Apply changes made
Recheck PATH Configuration: Verify that the Java binary is included in the PATH variable. Open the .bashrc or .bash_profile file again and add the following line:
export PATH="$PATH:$JAVA_HOME/bin"
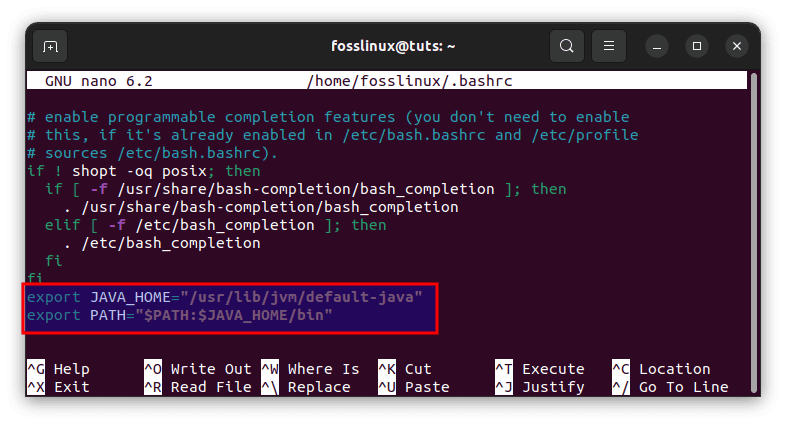
Append the following line
Save the file and execute the command:
source ~/.bashrc
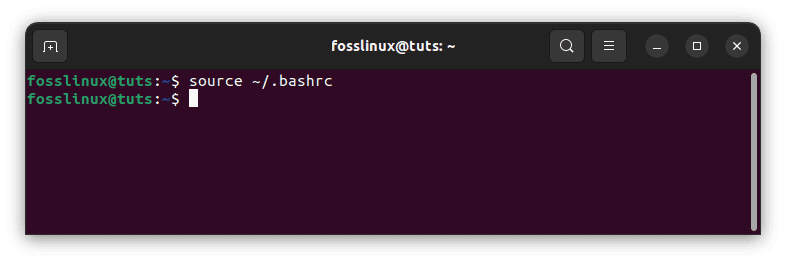
Apply changes made
Try running java -version again to check if the issue is resolved.
2. Classpath issues
You may encounter classpath-related issues when your Java program relies on external libraries or classes that are not in the default classpath. Here’s how to resolve them:
Specifying Classpath during Compilation: If your program depends on external libraries during compilation, use the -cp or -classpath option with the javac command. For example:
javac -cp path/to/library.jar MyClass.java
This command tells the compiler to include the specified JAR file (library.jar) in the classpath while compiling MyClass.java.
javac -cp /home/fosslinux/myapp.jar FossLinux.java
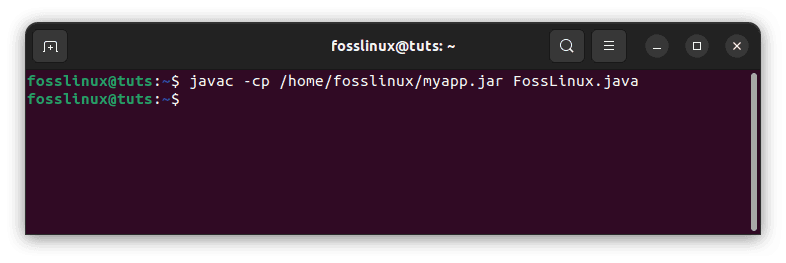
Specify classpath during compilation
Specifying Classpath during Execution: If your program requires additional classes or libraries at runtime, use the -cp or -classpath option with the java command. For example:
java -cp path/to/library.jar MyClass
This command ensures that the required classes from the specified JAR file are included in the classpath during program execution.
java -cp /home/fosslinux/myapp.jar FossLinux
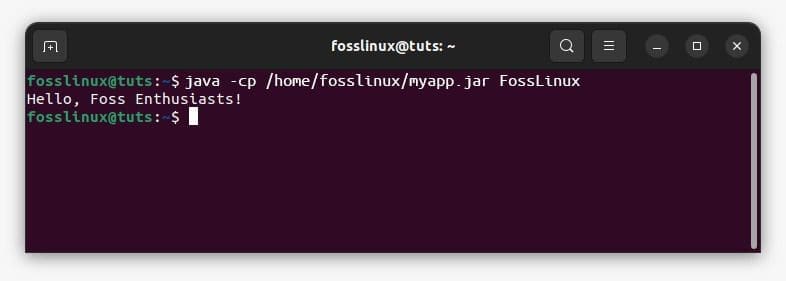
Specify classpath during execution
Using Classpath Wildcards: If you have multiple JAR files or directories containing classes, you can use wildcards (*) to simplify the classpath specification. For example:
java -cp path/to/libs/* MyClass
This command includes all JAR files in the “libs” directory in the classpath.
java -cp /home/fosslinux/* FossLinux
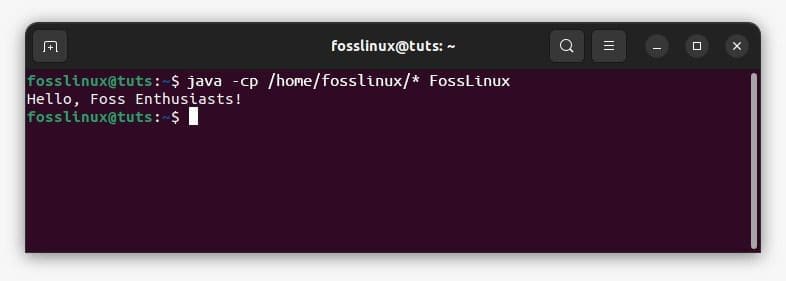
Use classpath wildcards
Exploring Alternative Build Tools: Consider using build tools like Maven or Gradle, which manage dependencies and automatically handle classpath configuration for you. These tools simplify the process of compiling and running Java programs that require external libraries.
3. Compilation Errors
While compiling Java programs, you may encounter errors due to syntax issues or missing dependencies. Here are some troubleshooting tips:
Syntax Errors: Carefully review your code for syntax errors, such as missing semicolons, mismatched parentheses, or incorrect variable declarations. The compiler will provide error messages that can help pinpoint the issue. Double-check your code against Java language specifications or consult relevant documentation.
Missing Dependencies: If your program relies on external libraries, ensure that the required JAR files are included in the classpath during compilation. If a compilation error occurs due to missing dependencies, follow these steps:
- Check Dependency Documentation: Refer to the documentation of the library or framework you are using to identify the required dependencies and their versions.
- Download and Include Dependencies: Download the necessary JAR files for the dependencies and place them in a designated directory, such as a “libs” folder within your project. Then, include these dependencies in the classpath during compilation using the -cp or -classpath option with the javac command. For example:
javac -cp path/to/libs/* MyClass.java
- Resolve Package Imports: If your code includes package imports that cannot be resolved, it may indicate that the required dependencies are not correctly included in the classpath. Double-check the package names and ensure that the corresponding JAR files are in the classpath during compilation and execution.
4. Debugging Java Programs
When encountering runtime errors or unexpected behavior in your Java program, you can use command-line tools to aid in debugging:
Stack Traces: When an exception occurs, Java provides a stack trace that shows the sequence of method calls leading up to the exception. This information can help pinpoint the location of the error. Analyze the stack trace and identify the line numbers mentioned to locate the issue in your code.
Debugging with jdb: The Java Debugger (jdb) is a command-line tool allowing you to interactively debug your Java programs. You can set breakpoints, step through code execution, inspect variables, and more. To use jdb, compile your Java program with the -g option to include debugging information, and then execute it using jdb followed by the name of the main class. For example:
javac -g MyClass.java jdb MyClass
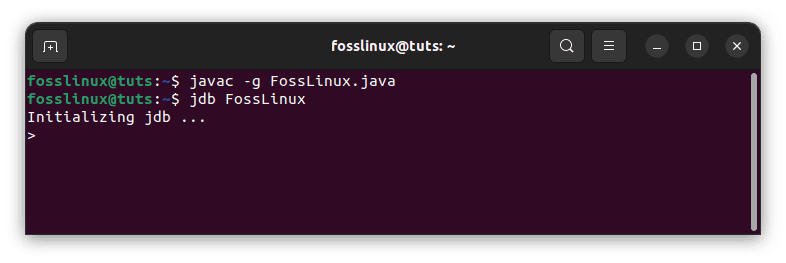
Debugging with jdb
Logging and Print Statements: Incorporate logging frameworks (such as Log4j or SLF4J) or print statements strategically within your code to output relevant information during runtime. These can provide valuable insights into the program’s execution flow and variable values, assisting in identifying and troubleshooting issues.
Remember, debugging is an iterative process. Analyze error messages, leverage available tools, and thoroughly examine your code to identify and resolve issues efficiently.
Helpful tips
Below are a few additional pieces of information that may be helpful for readers who want to run Java from the command line in Linux.
- Firstly, it is worth noting that the methods for running Java from the command line discussed in this article are not the only methods available. For example, you can use tools like Apache Maven or Gradle to manage and build Java projects from the command line. These tools can be handy for managing more significant projects with multiple dependencies.
- Additionally, it is worth noting that the installation process for Java on Linux may vary depending on the specific distribution and version that you are using. The methods outlined in this article are intended to be general guides. Still, referring to the documentation for your specific distribution would be best to ensure you install Java correctly.
- Finally, it is worth emphasizing the importance of keeping your Java installation up-to-date. Oracle regularly releases security updates and bug fixes for Java. Staying on top of these updates is crucial to ensure your Java installation remains secure and stable. Many Linux distributions provide tools for automatically managing software updates, so configure these tools to keep your Java installation up-to-date.
By keeping this additional information in mind, you can ensure that you can effectively run Java from the command line in Linux and stay up-to-date with the latest Java developments.
Conclusion
Congratulations on acquiring a comprehensive understanding of running Java programs from the command line in a Linux environment. This guide has covered the installation process, compiling and executing Java code, configuring environment variables, and troubleshooting common issues. By harnessing the command line, you now possess the flexibility and control to run Java programs efficiently.
Remember to approach troubleshooting systematically, verifying Java installation, checking environment variables, resolving classpath issues, and leveraging debugging tools when necessary. By practicing these techniques, you can overcome challenges and streamline your Java development workflow.
Thank you for joining us on this journey through the intricacies of running Java from the command line in Linux. We hope this guide has equipped you with the knowledge and tools necessary to confidently tackle Java projects. So embrace the power of the command line and continue exploring the vast possibilities of Java development. Happy coding!