Conditional statements are the bread and butter of programming. They allow the machine to make decisions procedurally, through a process of elimination and repetition, almost making it act like it has its brain. Just a brain with a minimal amount of knowledge and no creative prowess (yet!) but functioning, nevertheless.
Today, we will talk about one specific conditional statement: the if-else statement. We will show you how to write the syntax for Bash scripting and use its different variations.
Bash If-Else introduction
Logic
The logic of the if-else statement is quite simple: if a specific condition is met, you want your system to do a particular task, and if it is not, then you want it to do another. For example, if the age of a person is more than or equal to 18, you want them to know they are an adult and, conversely, if not, tell them that they are not an adult. In pseudo-code, that would look like:
if age is more than or equal to 18: display "You are an adult." if age is less than 18: display "You are not an adult yet."
The logic is sound, yes?
Syntax
The syntax for an if-else statement, in the case of Bash, looks like this:
if [ condition ] then //command if condition is true else //command if condition is false fi
Unlike Python, the indentation is not an essential part of the syntax here. It doesn’t depict the code inside the if block. The if statement block begins at the keyword “if” and ends at “fi”.
If-Else statement
Let us see the example in which we used logic in proper code. First, we need to create a file to write the code. I am going to go ahead and name it ifelse.sh:
nano ifelse.sh
The code is available below for copying, but I would suggest that you type it in yourself to get practice with the syntax:
age=20 if [ $age -ge 18 ] then echo "You are an adult." else echo "You are not an adult yet."
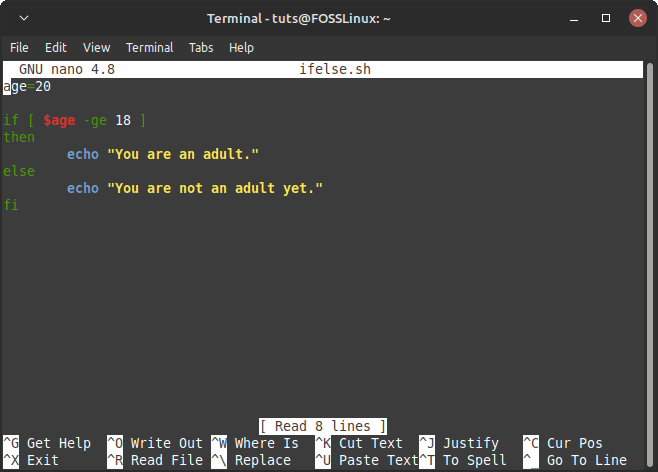
If Else Statement Example
Here are a few things to unpack here:
If you want to refer to a variable inside a script, you need to use the “$” symbol before the variable name. This is the case for all Linux variables, including the environment variables. For example:
echo $HOME
This tells you the location of your home directory.
Also, the one spacebar gap after the opening square bracket ‘[‘ and before the closing square bracket ‘]’ is significant for the syntax. If you don’t leave the gaps, the script will make some errors.
Next, the comparisons between numbers in Bash scripting can be made with notations like the one I used in the script.
Comparison | Description | Usual Mathematical Notation |
---|---|---|
-lt | Less Than | < |
-le | Less Than or Equal To | <= |
-gt | Greater Than | > |
-ge | Greater Than or Equal To | >= |
-eq | Equal To | !ERROR! C6 -> Formula Error: Unexpected operator '=' |
-ne | Not Equal To | != |
Execute this script with this command:
bash ifelse.sh
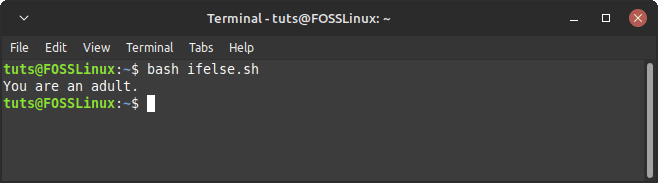
Simple if-else Script Execution
Since we set the age as 20, we get the expected result.
Now, in other programming languages, or even simple math, you can use the symbol “>” to denote greater than or “<” to denote less than comparisons. If we try that here, we get this error:
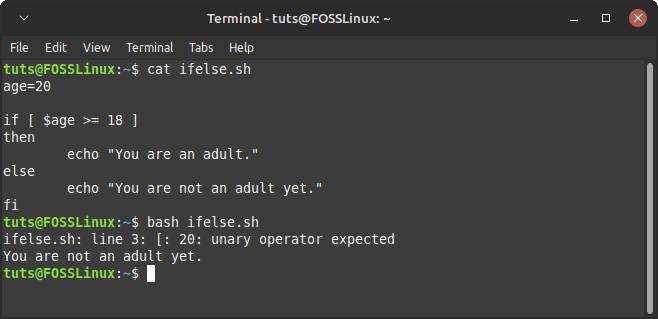
Modified Operator Error
This is because in Bash “>” is used to write the output of a command to a file. For example, if you run this command:
ls > lsresult
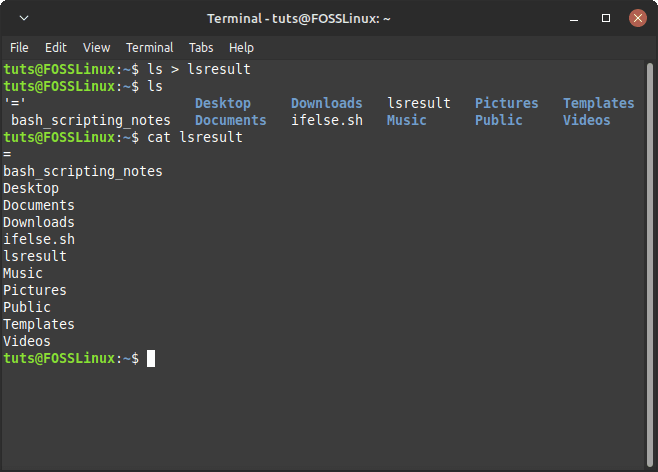
Write to File Example
The result of ls has been written to a file named “lsresult”. If you notice, there is a file called “=” because of our modified script earlier.
So how do you resolve this? You replace the square brackets around the command with two parentheses. That will make the shell interpret the statement as a simple comparison. The other comparisons denoted by the mathematical symbols mentioned in the third column of the table above can be made this way.
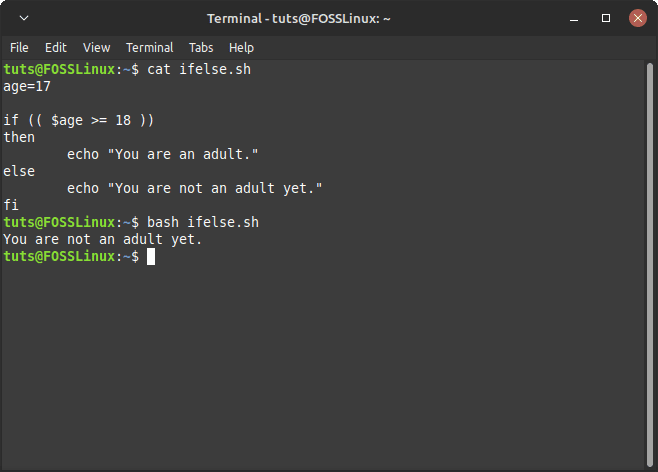
Correctly Modified Operator
If-Elif-Else Statement
The logic of the if-elif-else statement is based on multiple conditions. If there are numerous conditions that you need to check for, all independent of each other, you can add the ‘elif’ statement to make that happen.
Extending on the previous example, let us say that the age being eligible to get a driver’s license is 16. Now we modify our script in the following manner:
if age is less than 16: display "You are not an adult, nor can you get a driver's license." else if age is less than 18: display "You are not an adult, but you can get a driver's license." else: display "You are an adult and you can get a driver's license."
Here, we will also use a method of getting user input while executing the command. For user input after running the script, the read keyword is used with the -p flag, which denotes the requirement of a prompt. The code would look like this:
read -p "Enter your age: " age if [ $age -lt 16 ] then echo "You are not an adult, nor can you get a driver's license." elif [ $age -lt 18 ] then echo "You are not an adult, but you can get a driver's license." else echo "You are an adult and you can get a driver's license." fi
There is not much to explain here, except for the new read statement that gets user input and the all-new elif statement (the logic has already been explained). Now execute the script for different ages:
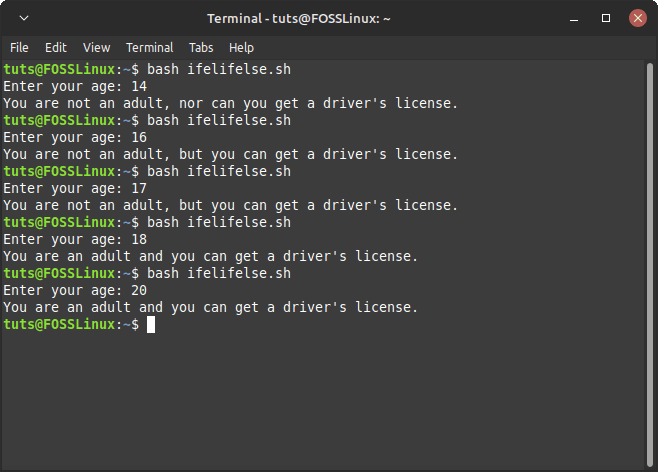
If-Elif-Else Example
Something is interesting going on here. If you think about it, the age of 14 satisfies both the “if” condition of being under 16 and the “elif” condition of being under 18. Why, then, are not both the commands executed? That’s because whichever condition in the serial sequence is satisfied first is considered the final one, and then if the block is not explored any further. While this works well, the logic of it is pretty messy. If you want a more clean code on your conscience, the next section of nested if statements will suit you better.
Nested If statement
Nested if loops are the ones that have an if conditional block inside of another if conditional block. This is better explained with an example. We will take the same example as above but with a nested loop.
We want first to check if the user is eligible to own a driver’s license or not, and then we want to check if the user is an adult.
If the age is less than 16: Display "You cannot get a driver's license nor are you an adult." Else if the age is greater than or equal to 16: If the age is less than 18: Display "You can get a driver's license but you are not an adult." Else if the age is greater than or equal to 18: Display "You can get a driver's license and you are an adult as well."
Now see what we did in the pseudo-code? There’s an if condition inside of an if condition. Here’s what the code looks like:
read -p "Enter your age: " age if [ $age -lt 16 ] then echo "You cannot get a driver's license nor are you an adult." elif [ $age -ge 16 ] then if [ $age -lt 18 ] then echo "You can get a driver's license but you are not an adult." elif [ $age -ge 18 ] then echo "You can get a driver's license and you are an adult as well." fi fi
You will notice that we have indented the code according to the level of depth of the written statements. The inner if block is indented one more step than the outer if block. While this is not necessary, it is certainly recommended. Another thing to see here is that we have entirely left out the else statement here. If your if and elif statements can cover everything, adding an else statement to your code is unnecessary.
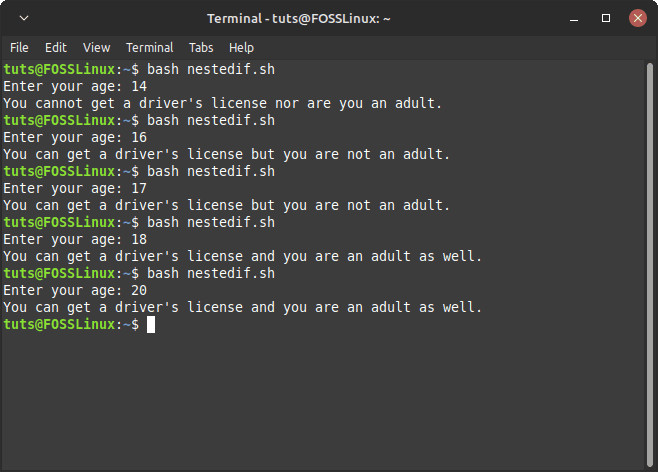
Nested If Example
Contrasting how we previously handled this example, this leaves no room for error. One age can only satisfy one condition, and it’s much cleaner this way. But what if you’re not a fan of all this layering? Well, we have a solution for you, too.
Multiple conditions
We will show you how to do that example in yet another way. This time, it is the cleanest and the shortest way. If you think about the example, there are three categories of people, correct?
Category 1: People who are below 16. You can’t get a driver’s license; you aren’t an adult either.
Category 2: People who are older than 16 but younger than 18. They can get a driver’s license, but not adults.
Category 3: People who are older than 18. They can get a driver’s license, also are adults.
So, in that case, the code becomes:
read -p "Enter your age: " age if [ $age -lt 16 ] then echo "You cannot get a driver's license, nor are you an adult." elif [ $age -ge 16 ] && [ $age -lt 18 ] then echo "You can get a driver's license but you are not an adult." elif [ $age -ge 18 ] then echo "You can get a driver's license and you are an adult." fi
The key player here is the ‘&&’ part. The ‘&&’ denotes the condition of AND. So it means, if the age is greater than or equal to 16 AND it is less than 18, the second condition is fulfilled. This leaves no overlapping room, as we saw in the case of the first execution of this example, nor does this require multiple layers, as we saw in the usage of the nested loops.
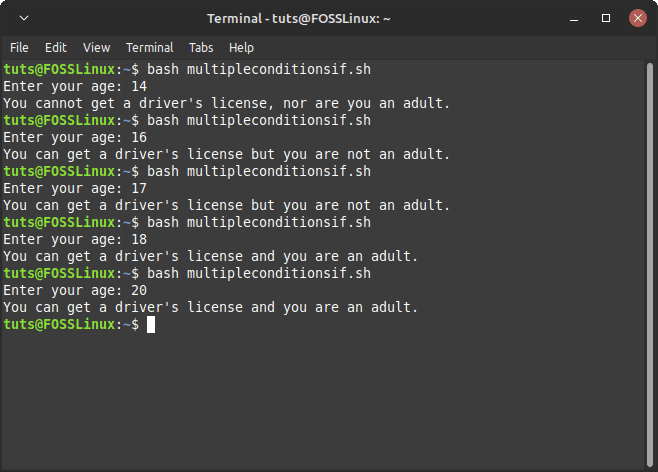
Multiple Conditions If-Else Statement
There is also an OR condition denoted by ‘||’. If you are using that, if any one of the conditions on either side of the OR is true, the condition is fulfilled, and the commands following it will be executed.
String Comparisons
So far, we have been comparing numbers. But what if it is strings (words) that you need to compare? There’s a bit of an explanation to consider when comparing strings. The equality is apparent; if both the strings are identical, they are equal. If they are not similar, then they are not equal.
The lesser than and greater than comparisons are based on alphabetical order. It will be smaller if the first different alphabet in the sequence comes first in alphabetical order. For example, “Abel” is smaller than “Adel” because B comes before D in alphabetical order. Here, there is no case sensitivity, but different cases will make the strings unequal in the case of equality of strings.
Now create a script for the strings mentioned above:
str1="Abel" str2="Adel" if [[ $str1 > $str2 ]] then echo "$str1 is greater than $str2." else echo "$str1 is less than $str2." fi
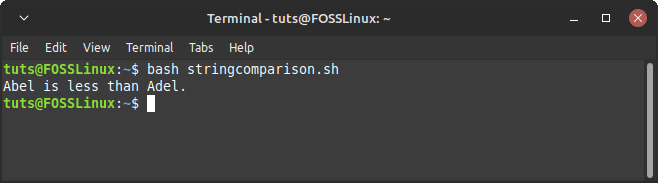
If-Else String Comparison
If you notice here, we have used double square brackets instead of the single one in the above examples. This is about the problem mentioned before about “>” being used to write to a file. The double brackets ensure only comparison happens inside them.
Case Statement
This is not a part of the if-elif-else structure, but this has a similar purpose. The case statement is helpful for a rather large group of possible outcomes. For example, you are an employer who more than 10 employees. You have different tasks for different groups of those employees. You want Casey, Ben, and Matt to send out invitations. You want Rachel, Amy, and Jill to set up shop equipment (yes, that’s a Friends reference), and you want Harry, Hermione, Ron, and Ginny to attend to the customers (yes, yes, of course, that’s a Harry Potter reference). For anyone else, you want them to ask the administrator what to do. You can either do this with really long if commands or use the case command. The structure of the case command looks like this:
case variable in pattern1 | pattern2) commands1;; pattern3) commands2;; *) commands3;; esac
As in the if statement, the case block ends with “esac”. Also, the asterisk (*) acts as a wildcard character, just like in the Linux command line. It means everything. If you write “B*,” this would denote everything that starts with a “B”. Now let’s implement our example in code.
read -p "Enter your name: " name case $name in Casey | Ben | Matt) echo "$name, your job is to send out invitations.";; Rachel | Amy | Jill) echo "$name, your job is to set up shop equipment.";; Harry | Hermione | Ron | Ginny) echo "$name, your job is to attend to the customers.";; *) echo "$name, please report to the administrator to know your task.";; esac
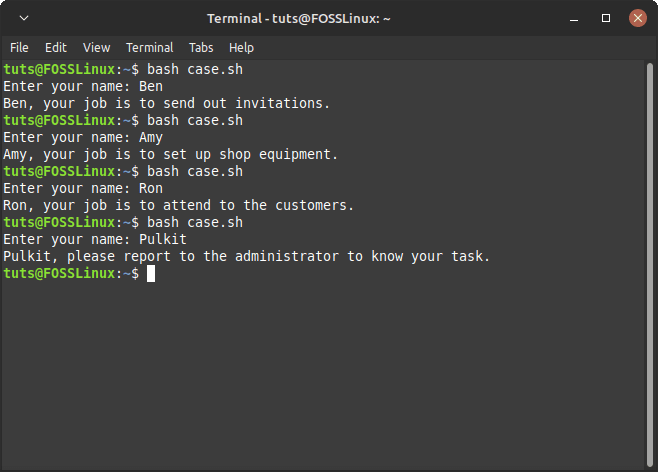
Case Statement Example
If you tried to do this with an if statement, that would involve a lot of OR conditions. That’s usually not considered good practice. Case statement is recommended in those cases, as long as you get the syntax right.
Conclusion
This is but one part of the big world of Bash scripting. As you would expect from a programming language, it contains options of all kinds spanning multiple use cases. But as mentioned before, this is one of the essential things in all programming because conditional statements are the backbone of making a computer understand basic logic. We hope that this article was helpful to you.